C++ Exercises: Display the sum of the series 1+x+x^2/2!+x^3/3!+....
C++ For Loop: Exercise-24 with Solution
Write a program in C++ to display the sum of the series [ 1+x+x^2/2!+x^3/3!+....].
Sample Solution:
C++ Code :
#include <iostream> // Including input-output stream header
using namespace std; // Using standard namespace to avoid writing std::
int main() // Start of the main function
{
float x, sum, no_row; // Declaration of float variables 'x' (input value), 'sum' (to store the sum), 'no_row' (value of the current term)
int i, n; // Declaration of integer variables 'i' (loop counter), 'n' (number of terms)
cout << "\n\n Display the sum of the series [ 1+x+x^2/2!+x^3/3!+....]\n"; // Displaying a message on the console
cout << "------------------------------------------------------------\n";
cout << " Input the value of x: "; // Prompting the user to input the value of x
cin >> x; // Reading the input value as 'x'
cout << " Input number of terms: "; // Prompting the user to input the number of terms
cin >> n; // Reading the input value as the number of terms
sum = 1; // Initializing 'sum' with the first term of the series (1)
no_row = 1; // Initializing 'no_row' with the first term (1)
for (i = 1; i < n; i++) // Loop to calculate 'n' terms of the series
{
no_row = no_row * x / (float)i; // Calculating the value of the current term based on the formula
sum = sum + no_row; // Adding the current term to the sum
}
cout << " The sum is : " << sum << endl; // Displaying the sum of the series
}
Sample Output:
Display the sum of the series [ 1+x+x^2/2!+x^3/3!+....] ------------------------------------------------------------ Input the value of x: 3 Input number of terms: 5 The sum is : 16.375
Flowchart:
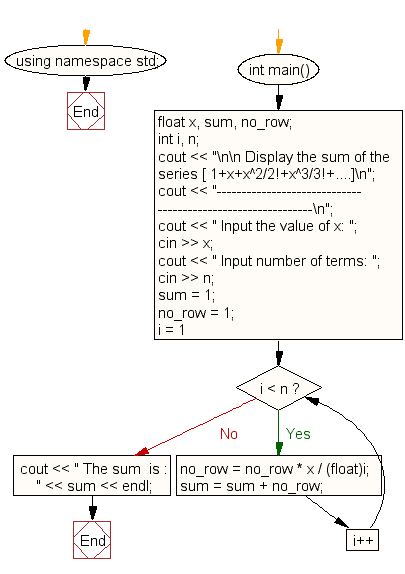
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the sum of the series [ 9 + 99 + 999 + 9999 ...].
Next: Write a program in C++ to find the sum of the series [ x - x^3 + x^5 + ......].
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics