C++ Exercises: Display Pascal's triangle like pyramid
45. Pascal's Triangle as Pyramid
Write a C++ program to display Pascal's triangle like a pyramid.
Construction of Pascal's Triangle:
As shown in Pascal's triangle, each element is equal to the sum of the two numbers immediately above it.
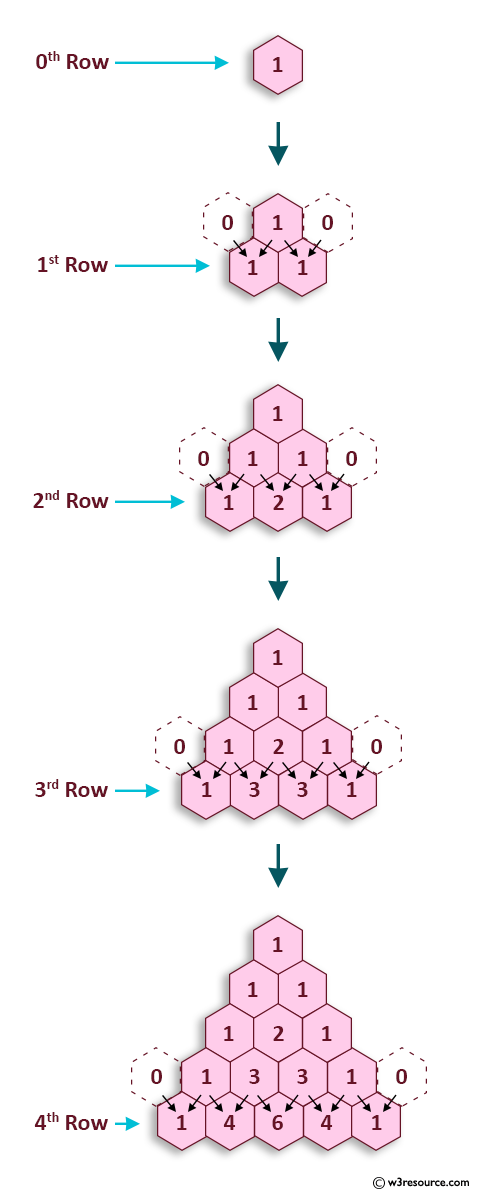
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int row, c = 1, blk, i, j; // Declare integer variables row, c, blk, i, and j
// Display message asking for input
cout << "\n\n Display the Pascal's triangle:\n";
cout << "-----------------------------------\n";
cout << " Input number of rows: ";
cin >> row; // Read input for the number of rows from the user
for (i = 0; i < row; i++) // Loop for the number of rows
{
for (blk = 1; blk <= row - i; blk++) // Loop to print spaces before the numbers
cout << " "; // Print two spaces for formatting
for (j = 0; j <= i; j++) // Loop to calculate and print the numbers in each row
{
if (j == 0 || i == 0) // Check if it's the first column or the first row
c = 1; // Assign 1 to 'c' if it's the first column or the first row
else
c = c * (i - j + 1) / j; // Calculate the next number using the previous value
cout << c << " "; // Print the calculated number followed by spaces for formatting
}
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Display the Pascal's triangle: ----------------------------------- Input number of rows: 5 1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
Flowchart:
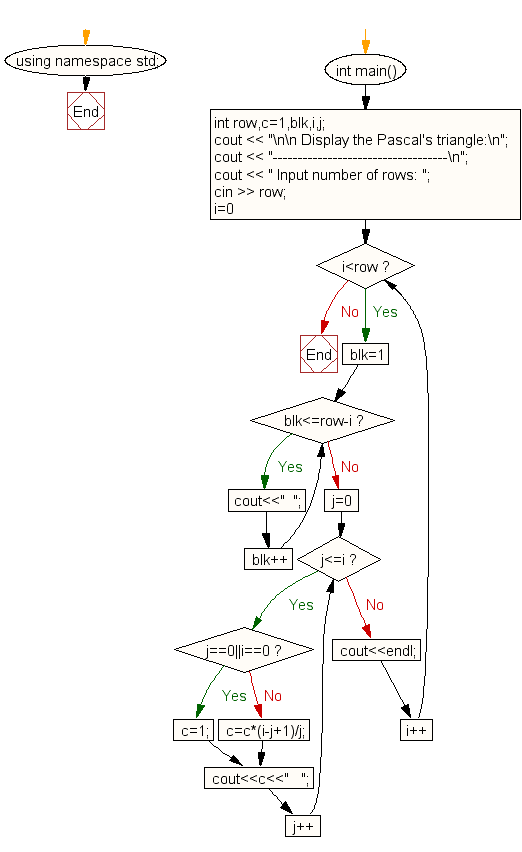
For more Practice: Solve these Related Problems:
- Write a C++ program to generate Pascal's Triangle in a centered pyramid format using nested loops.
- Write a C++ program that reads the number of rows and prints Pascal's Triangle with proper spacing to form a pyramid.
- Write a C++ program to display Pascal's Triangle as a pyramid, formatting each number with fixed width for alignment.
- Write a C++ program to construct Pascal's Triangle and output it in a pyramid shape, using recursion to compute binomial coefficients.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the pattern like a diamond.
Next: Write a program in C++ to display Pascal's triangle like right angle triangle.
What is the difficulty level of this exercise?