C++ Exercises: Display the pattern like a diamond
44. Diamond Pattern
Write a C++ program to display a pattern like a diamond.
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, r; // Declare integer variables i, j, and r
// Display message asking for input
cout << "\n\n Display the pattern like a diamond:\n";
cout << "----------------------------------------\n";
cout << " Input number of rows (half of the diamond): ";
cin >> r; // Read input for the number of rows (half of the diamond) from the user
// Loop to print the upper half of the diamond pattern
for (i = 0; i <= r; i++) // Loop for the upper half of the diamond
{
for (j = 1; j <= r - i; j++) // Loop to print spaces before the asterisks
{
cout << " "; // Print a space
}
for (j = 1; j <= 2 * i - 1; j++) // Loop to print asterisks in each row
{
cout << "*"; // Print an asterisk
}
cout << endl; // Move to the next line after each row is printed
}
// Loop to print the lower half of the diamond pattern
for (i = r - 1; i >= 1; i--) // Loop for the lower half of the diamond
{
for (j = 1; j <= r - i; j++) // Loop to print spaces before the asterisks
{
cout << " "; // Print a space
}
for (j = 1; j <= 2 * i - 1; j++) // Loop to print asterisks in each row
{
cout << "*"; // Print an asterisk
}
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Display the pattern like a diamond: ---------------------------------------- Input number of rows (half of the diamond): 5 * *** ***** ******* ********* ******* ***** *** *
Flowchart:
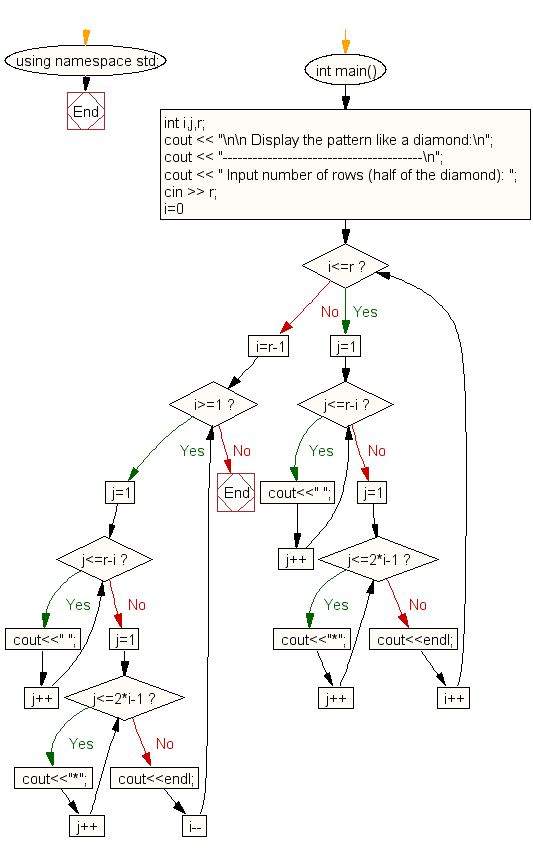
For more Practice: Solve these Related Problems:
- Write a C++ program to print a diamond pattern using '*' characters by first printing an increasing pyramid then a decreasing pyramid.
- Write a C++ program to generate a diamond pattern where the upper half is constructed normally and the lower half is its mirror image.
- Write a C++ program to display a diamond pattern with odd numbers at each level instead of asterisks, maintaining symmetry.
- Write a C++ program that prints a diamond shape where the number of stars increases then decreases, and each row is centered with spaces.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to print the Floyd's Triangle.
Next: Write a program in C++ to display Pascal's triangle like pyramid.
What is the difficulty level of this exercise?