C++ Exercises: Display the pattern power of 2, triangle
C++ For Loop: Exercise-54 with Solution
Write a program in C++ to display the pattern like pyramid, power of 2.
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
#include <math.h> // Include math library for pow() function
using namespace std; // Using standard namespace
int main() // Main function where the execution of the program starts
{
int i, j, spc, n, mm, d = 1, k; // Declare integer variables i, j, spc, n, mm, d, k
cout << "------------------------------------------------------\n"; // Display a separator line
cout << " Input the number of rows: ";
cin >> n; // Read input for the number of rows from the user
// Loop to print the first line (for the value 2^0)
for (i = 1; i <= n * 2 + 2 + 5; i++) // Extra spaces for margin from the left
cout << " "; // Print spaces for formatting
cout << pow(2, 0) << endl; // Print the value 2^0 followed by a newline
for (i = 1; i < n; i++) // Loop for the remaining lines
{
// Loop to print spaces before the numbers
for (k = 1; k <= n * 2 - d + 5; k++) // Extra spaces for margin from the left
{
cout << " "; // Print spaces for formatting
}
// Loop to print numbers up to the middle
for (j = 0; j < i; j++) // Loop from 0 to i
{
mm = pow(2, j); // Calculate the value 2 raised to the power of j
cout << mm << " "; // Print the calculated value followed by two spaces
}
// Loop to print numbers after the middle to the end
for (j = i; j >= 0; j--) // Loop from i to 0
{
mm = pow(2, j); // Calculate the value 2 raised to the power of j
cout << mm << " "; // Print the calculated value followed by two spaces
}
d = d + 3; // Increment the value of 'd' by 3 for formatting
cout << endl; // Move to the next line after each row is printed
}
}
Sample Output:
Input the number of rows: 5 1 1 2 1 1 2 4 2 1 1 2 4 8 4 2 1 1 2 4 8 16 8 4 2 1
Flowchart:
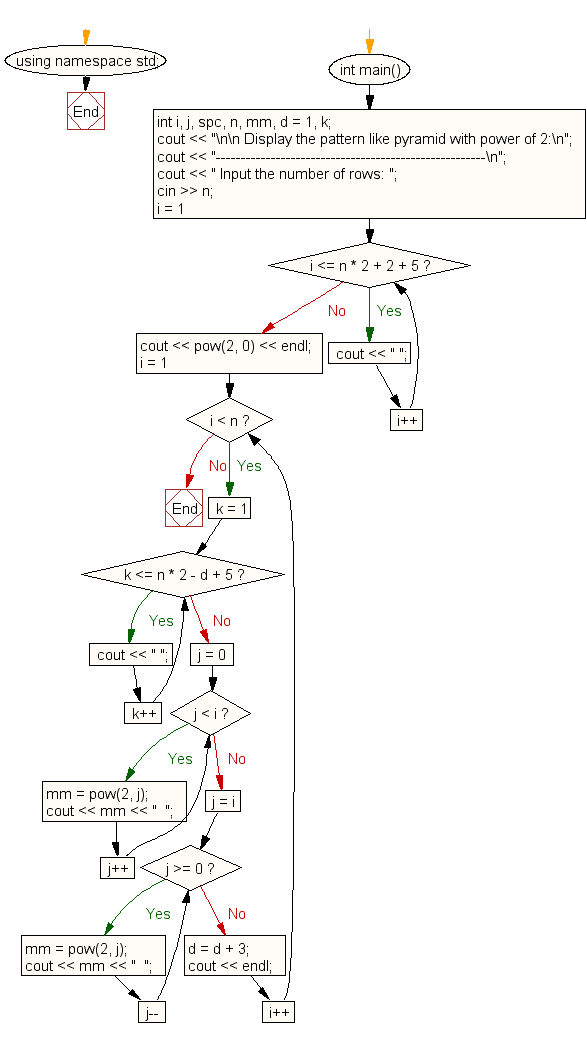
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the pattern like right angle triangle with right justified using digits.
Next: Write a program in C++ to display such a pattern for n number of rows using number. Each row will contain odd numbers of number.
The first and last number of each row will be 1 and middle column will be the row number. n numbers of columns will appear in 1st row.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/for-loop/cpp-for-loop-exercise-54.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics