C++ Exercises: Convert a octal number to binary number
77. Octal to Binary Conversion
Write a C++ program to convert an octal number to a binary number.
Visual Presentation:
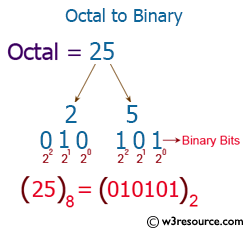
Sample Solution:-
C++ Code :
#include <iostream> // Include input/output stream library
#include <math.h> // Include math library for mathematical functions
using namespace std; // Use the standard namespace
int main() // Main function where the execution of the program starts
{
// Array representing binary values for octal digits from 0 to 7
int octal_numvalues[] = {0, 1, 10, 11, 100, 101, 110, 111};
// Declare variables for octal, temporary octal, binary number, remainder, and place value
long octal_num, tempoctal_num, binary_num, place;
int rem;
cout << "\n\n Convert any octal number to binary number:\n"; // Display message prompting for octal to binary conversion
cout << "------------------------------------------------\n"; // Display separator line
cout << " Input any octal number: "; // Prompt user to input an octal number
cin >> octal_num; // Read the octal number input by the user
tempoctal_num = octal_num; // Store the original octal number for reference
binary_num = 0; // Initialize the binary number
place = 1; // Initialize the place value for binary conversion
// Loop to convert octal to binary using array mapping
while (tempoctal_num != 0)
{
rem = (int)(tempoctal_num % 10); // Get the remainder by dividing the octal number by 10
binary_num = octal_numvalues[rem] * place + binary_num; // Convert octal digit to its binary equivalent and add to the result
tempoctal_num /= 10; // Remove the processed digit from the octal number
place *= 1000; // Increment the place value for the next binary digit position
}
cout << " The equivalent binary number: " << binary_num << "\n"; // Display the equivalent binary number
}
Sample Output:
Convert any octal number to binary number: ------------------------------------------------ Input any octal number: 17 The equivalent binary number: 1111
Flowchart:
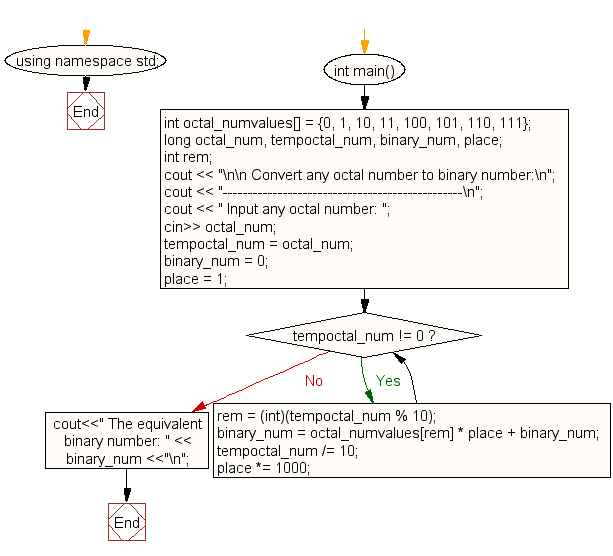
For more Practice: Solve these Related Problems:
- Write a C++ program to convert an octal number to its binary representation by first converting to decimal then to binary.
- Write a C++ program that reads an octal number and outputs its binary equivalent by mapping each octal digit to a 3-bit binary string.
- Write a C++ program to compute the binary representation of an octal input using conversion tables for each digit.
- Write a C++ program that converts an octal number to binary by processing each digit and concatenating its binary form.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to convert a octal number to decimal number.
Next: Write a program in C++ to convert a octal number to a hexadecimal number.
What is the difficulty level of this exercise?