C++ Exercises: Compute the sum of the digits of an integer
83. Sum of the Digits of an Integer
Write a C++ program to compute the sum of the digits of an integer.
Visual Presentation:
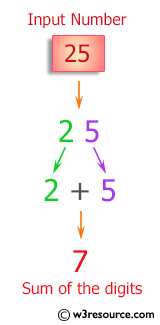
Sample Solution:-
C++ Code :
#include <iostream> // Including the input-output stream library
using namespace std; // Using standard namespace
int main() // Main function
{
int num1, sum, n; // Declaring three integer variables: num1, sum, n
sum = 0; // Initializing the variable 'sum' to zero
// Displaying a message to compute the sum of the digits of an integer
cout << "\n\n Compute the sum of the digits of an integer:\n";
cout << "-------------------------------------------------\n";
// Asking the user to input any number
cout << " Input any number: ";
cin >> num1; // Taking user input for 'num1'
n = num1; // Assigning the value of 'num1' to 'n' to preserve the original number
// Loop to compute the sum of the digits of the input number
while (num1 != 0)
{
sum += num1 % 10; // Adding the last digit of 'num1' to 'sum'
num1 /= 10; // Removing the last digit from 'num1'
}
// Displaying the sum of the digits of the original number
cout << " The sum of the digits of the number " << n << " is: " << sum << endl;
}
Sample Output:
Compute the sum of the digits of an integer: ------------------------------------------------- Input any number: 25 The sum of the digits of the number 25 is: 7
Flowchart:
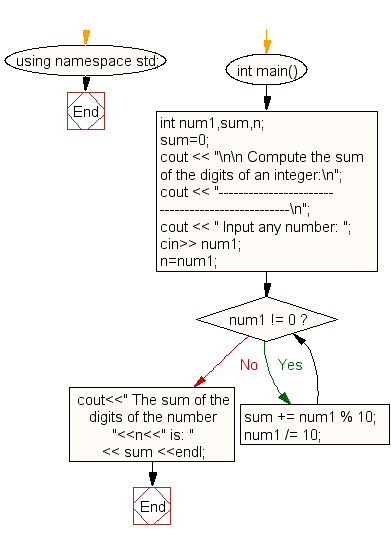
For more Practice: Solve these Related Problems:
- Write a C++ program to compute the sum of all digits in a given integer using a while loop.
- Write a C++ program that reads a number and calculates the sum of its digits by repeatedly extracting the last digit.
- Write a C++ program to sum the digits of an integer recursively and print the final result.
- Write a C++ program that converts an integer to a string and iterates over each character to sum its numeric value.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to compare two numbers.
Next: Write a program in C++ to compute the sum of the digits of an integer using function.
What is the difficulty level of this exercise?