C++ Linked List Exercises: Insert a new node at the beginning of a Linked List
4. Insert Node at the Beginning of Singly Linked List
Write a C++ program to insert a new node at the beginning of a Singly Linked List.
Visualization:
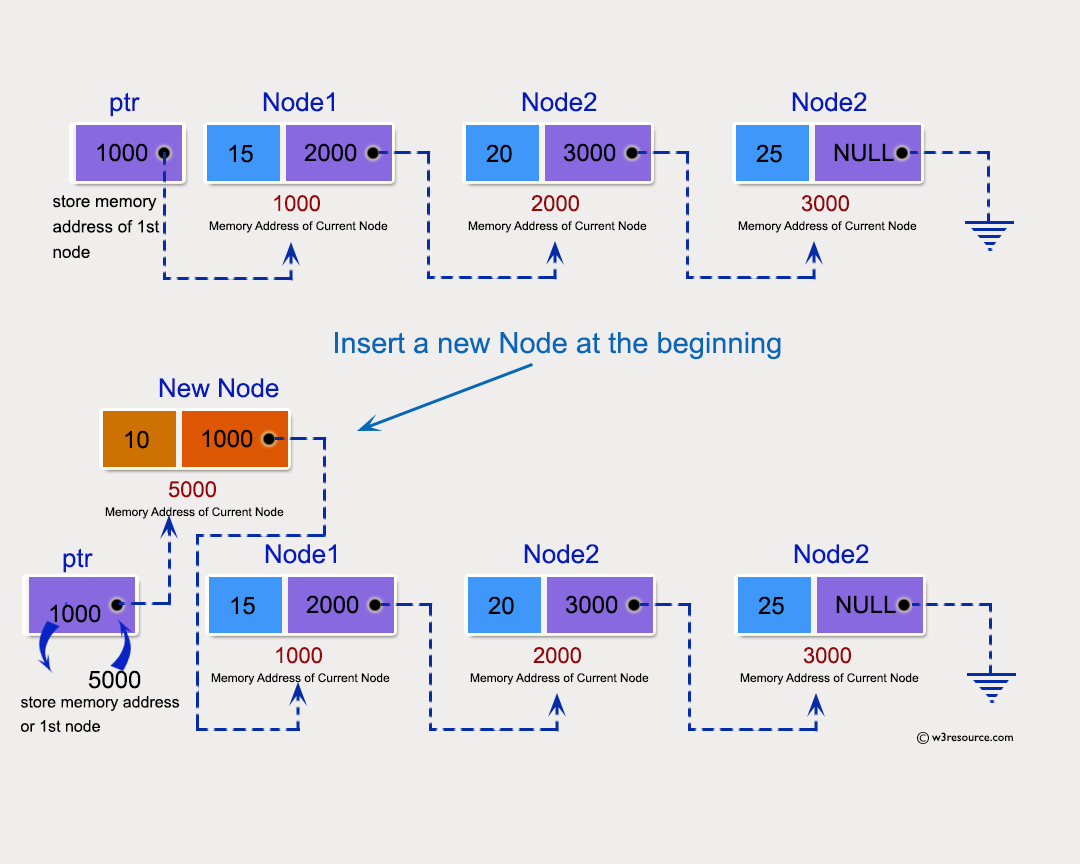
Test Data:
Original Linked list:
13 11 9 7 5 3 1
Insert a new node at the beginning of a Singly Linked List:
0 13 11 9 7 5 3 1
Sample Solution:
C++ Code:
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
//Declare node
struct Node{
int num; // Data field to store a number
Node *next; // Pointer to the next node
};
//Starting (Head) node
struct Node *head=NULL; // Initializing the head node as NULL initially
//Insert node at start
void insert_Node(int n){
struct Node *new_node=new Node; // Creating a new node
new_node->num=n; // Assigning data to the new node
new_node->next=head; // Pointing the new node to the current head
head=new_node; // Making the new node as the head
}
// Function to insert a new node at the beginning of the linked list
void NodeInsertatBegin(int new_element) {
// Create a new node
Node* new_Node = new Node();
new_Node->num = new_element; // Assigning data to the new node
new_Node->next = head; // Pointing the new node to the current head
head = new_Node; // Making the new node as the head
}
//Display all nodes
void display_all_nodes() {
struct Node *temp = head; // Temporary node to traverse the list
while(temp != NULL) { // Loop to iterate through the list
cout << temp->num << " "; // Displaying the data in the current node
temp = temp->next; // Moving to the next node
}
}
int main(){
insert_Node(1); // Inserting a node with value 1
insert_Node(3); // Inserting a node with value 3
insert_Node(5); // Inserting a node with value 5
insert_Node(7); // Inserting a node with value 7
insert_Node(9); // Inserting a node with value 9
insert_Node(11); // Inserting a node with value 11
insert_Node(13); // Inserting a node with value 13
cout << "Original Linked list:\n";
display_all_nodes(); // Displaying all nodes in the original list
cout << "\n\nInsert a new node at the beginning of a Singly Linked List:\n";
NodeInsertatBegin(0); // Inserting a new node with value 0 at the beginning
display_all_nodes(); // Displaying all nodes after insertion
cout << endl; // Displaying newline
return 0; // Returning from the main function
}
Sample Output:
Original Linked list: 13 11 9 7 5 3 1 Insert a new node at the beginning of a Singly Linked List: 0 13 11 9 7 5 3 1
Flowchart:
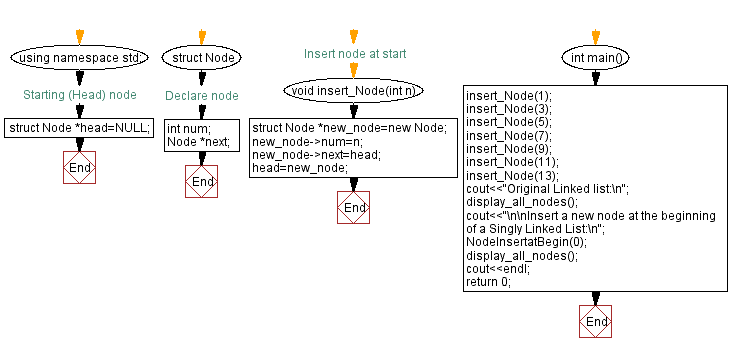
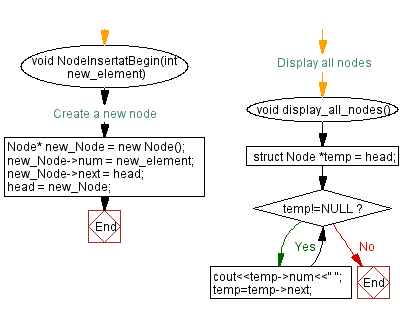
For more Practice: Solve these Related Problems:
- Develop a C++ program to insert multiple nodes at the beginning of a singly linked list and display the updated list.
- Write a C++ program that adds a node at the beginning and then verifies the insertion by printing the list with node indices.
- Design a C++ program to insert a new node at the beginning of a singly linked list and then display both the original and updated head addresses.
- Implement a C++ program to create a singly linked list, insert a node at the beginning, and then display the list in reverse order to confirm insertion.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Count number of nodes in a linked list.
Next C++ Exercise: Insert a new node at the end of a Linked List.
What is the difficulty level of this exercise?