C++ Linked List Exercises: Insert a new node at the end of a Linked List
5. Insert Node at the End of Singly Linked List
Write a C++ program to insert a new node at the end of a Singly Linked List.
Visualization:
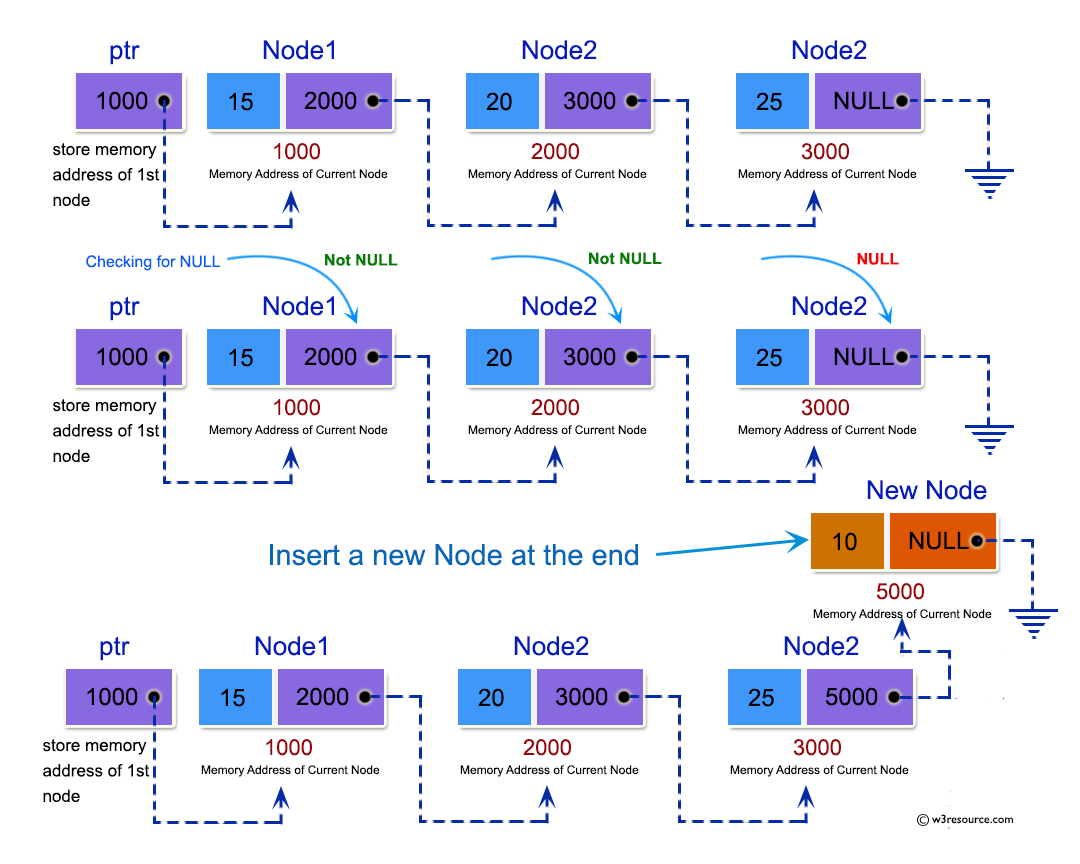
Test Data:
Original Linked list:
13 11 9 7 5 3 1
Insert a new node at the end of a Singly Linked List:
13 11 9 7 5 3 1 0
Sample Solution:
C++ Code:
#include <iostream> // Including input-output stream header file
using namespace std; // Using standard namespace
//Declare node
struct Node{
int num; // Data field to store a number
Node *next; // Pointer to the next node
};
//Starting (Head) node
struct Node *head=NULL; // Initializing the head node as NULL initially
//Insert node at start
void insert_Node(int n){
struct Node *new_node=new Node; // Creating a new node
new_node->num=n; // Assigning data to the new node
new_node->next=head; // Pointing the new node to the current head
head=new_node; // Making the new node as the head
}
// Function to insert a new node at the end of the linked list
void push_back(int newElement) {
Node* new_Node = new Node(); // Creating a new node
new_Node->num = newElement; // Assigning data to the new node
new_Node->next = NULL; // Initializing the next pointer of the new node to NULL
if(head == NULL) { // Checking if the linked list is empty
head = new_Node; // If empty, make the new node as the head
}
else {
Node* temp = head; // Temporary node to traverse the list
while(temp->next != NULL) // Loop to reach the last node
temp = temp->next; // Move to the next node
temp->next = new_Node; // Pointing the last node's next to the new node
}
}
//Display all nodes
void display_all_nodes() {
struct Node *temp = head; // Temporary node to traverse the list
while(temp != NULL) { // Loop to iterate through the list
cout << temp->num << " "; // Displaying the data in the current node
temp = temp->next; // Moving to the next node
}
}
int main(){
insert_Node(1); // Inserting a node with value 1
insert_Node(3); // Inserting a node with value 3
insert_Node(5); // Inserting a node with value 5
insert_Node(7); // Inserting a node with value 7
insert_Node(9); // Inserting a node with value 9
insert_Node(11); // Inserting a node with value 11
insert_Node(13); // Inserting a node with value 13
cout << "Original Linked list:\n";
display_all_nodes(); // Displaying all nodes in the original list
cout << "\n\nInsert a new node at the end of a Singly Linked List:\n";
push_back(0); // Inserting a new node with value 0 at the end of the list
display_all_nodes(); // Displaying all nodes after insertion
cout << endl; // Displaying newline
return 0; // Returning from the main function
}
Sample Output:
Original Linked list: 13 11 9 7 5 3 1 Insert a new node at the end of a Singly Linked List: 13 11 9 7 5 3 1 0
Flowchart:
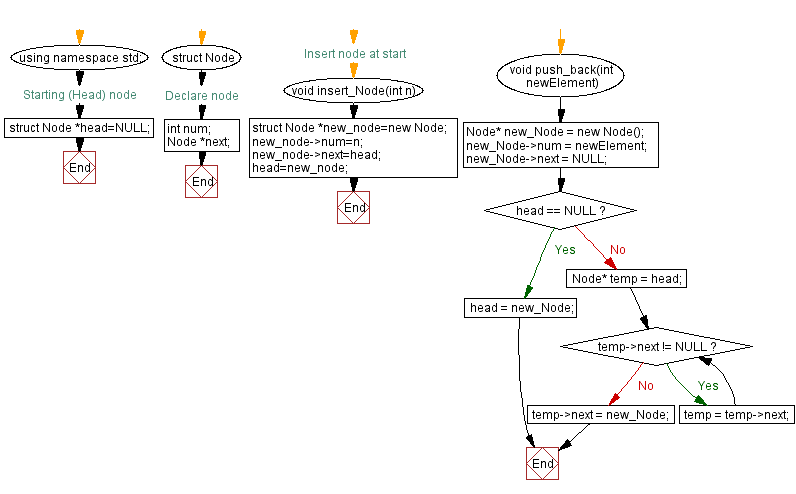
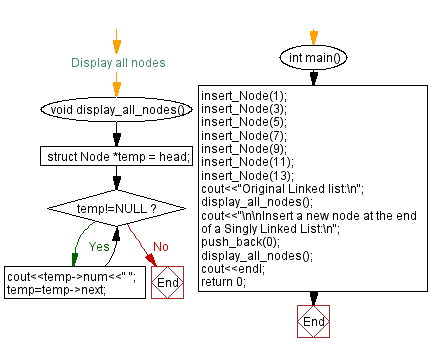
For more Practice: Solve these Related Problems:
- Write a C++ program to append a node at the end of a singly linked list and display the list using pointer traversal.
- Develop a C++ program to insert a node at the end of a singly linked list and then print the list along with the last node’s pointer.
- Design a C++ program that creates a singly linked list, appends a node with a random value at the end, and then displays the complete list.
- Implement a C++ program to add a new node at the end of a singly linked list and display the updated list using a do-while loop.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Insert a new node at the beginning of a Linked List.
Next C++ Exercise: Find middle element in a single linked list.
What is the difficulty level of this exercise?