C++ Exercises: Count the total number of digit 1 pressent in all positive numbers less than or equal to a given integer
25. Count Total Occurrences of Digit 1 in Numbers Up to n
Write a C++ program to count the total number of digits 1 present in all positive numbers less than or equal to a given integer.
Sample Input: n = 10
Sample Output: Number of digit 1 present in all +ve numbers less than or equal to 10 is 2
Sample Input: n = 19
Sample Output:
Number of digit 1 present in all +ve numbers less than or equal to 19 is 12
Sample Solution:
C++ Code :
#include <iostream>
#include <cmath>
using namespace std;
// Function to count the occurrence of digit 1 in positive integers up to 'n'
int count_digit_one(int n) {
int ctr = 0; // Initialize a counter for digit '1' occurrences
// Iterate through each digit place, starting from the ones place
for (int i = 1; i <= n; i *= 10) {
int a = n / i; // Get the quotient when dividing 'n' by 'i'
int b = n % i; // Get the remainder when dividing 'n' by 'i'
// Calculate the occurrences of digit '1' at each digit place and accumulate them
ctr += (a + 8) / 10 * i;
// Check if the current digit is '1' and add the count accordingly
if (1 == a % 10) {
ctr += b + 1;
}
}
return ctr; // Return the total count of digit '1' occurrences
}
int main() {
int n = 10;
// Display the count of digit '1' present in all positive numbers less than or equal to 'n'
cout << "Number of digit 1 present in all +ve numbers less than or equal to " << n << " is " << count_digit_one(n);
n = 19;
cout << "\nNumber of digit 1 present in all +ve numbers less than or equal to " << n << " is " << count_digit_one(n);
n = 100;
cout << "\nNumber of digit 1 present in all +ve numbers less than or equal to " << n << " is " << count_digit_one(n);
return 0;
}
Sample Output:
Number of digit 1 present in all +ve numbers less than or equal to 10 is 2 Number of digit 1 present in all +ve numbers less than or equal to 19 is 12 Number of digit 1 present in all +ve numbers less than or equal to 100 is 21
Flowchart:
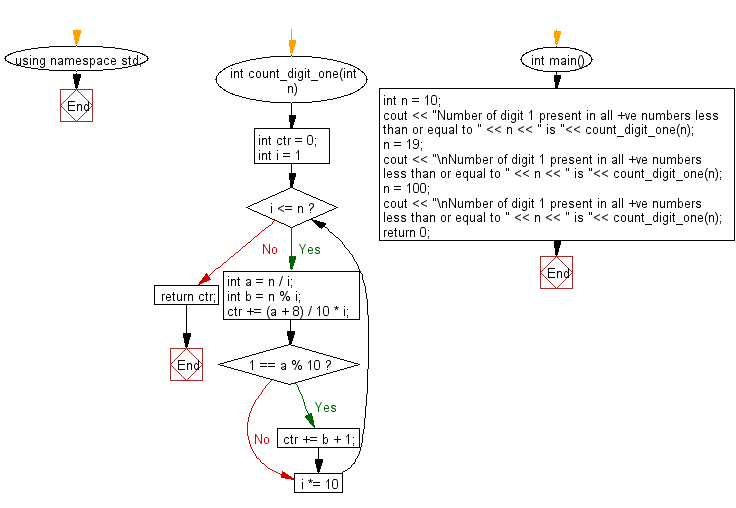
For more Practice: Solve these Related Problems:
- Write a C++ program to count the number of times digit 1 appears in all numbers from 1 to n by iterating through each number.
- Write a C++ program that computes the frequency of digit 1 in the range 1 to n using mathematical analysis of digit positions.
- Write a C++ program to recursively count occurrences of digit 1 in numbers up to n and return the total count.
- Write a C++ program that employs a combination of loops and modulus operations to determine the total count of digit 1 in all numbers up to n.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to count the prime numbers less than a given positive number.
Next: Write a C++ program to find the missing number in a given array of integers taken from the sequence 0, 1, 2, 3, ...,n.
What is the difficulty level of this exercise?