C++ Exercises: Number of arithmetic slices in an array of integers
C++ Math: Exercise-32 with Solution
Write a C++ program to find the number of arithmetic slices in a given array of integers.
From Wikipedia
In mathematics, an arithmetic progression (AP) or arithmetic sequence is a sequence of numbers such that the difference between the consecutive terms is constant. Difference here means the second minus the first. For instance, the sequence 5, 7, 9, 11, 13, 15, . . . is an arithmetic progression with common difference of 2.
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to count the number of arithmetic slices in an array
int number_Of_Arithmetic_Slices(int nums[], int arr_size) {
int sum = 0; // Variable to store the total number of arithmetic slices
int curr = 0; // Counter to track the current number of arithmetic slices
// Iterate through the array starting from the third element
for (int i = 2; i < arr_size; i++) {
// Check if the current three consecutive elements form an arithmetic sequence
if (nums[i] - nums[i - 1] == nums[i - 1] - nums[i - 2]) {
curr++; // Increment the current count of slices
sum += curr; // Add the current count to the total number of slices
} else {
curr = 0; // Reset the current count if the sequence breaks
}
}
return sum; // Return the total number of arithmetic slices
}
int main() {
// Example 1
int nums1[7] = {1, 2, 3, 9, 4, 5, 6};
int arr_size = sizeof(nums1) / sizeof(nums1[0]);
cout << "Original array: ";
for (int i = 0; i < arr_size; i++)
cout << nums1[i] << " ";
cout << "\nNumber of arithmetic slices: " << number_Of_Arithmetic_Slices(nums1, arr_size) << endl;
// Example 2
int nums2[4] = {1, 3, 5, 7};
arr_size = sizeof(nums2) / sizeof(nums2[0]);
cout << "\nOriginal array: ";
for (int i = 0; i < arr_size; i++)
cout << nums2[i] << " ";
cout << "\nNumber of arithmetic slices: " << number_Of_Arithmetic_Slices(nums2, arr_size) << endl;
// Example 3
int nums3[5] = {2, 1, 3, 4, 7};
arr_size = sizeof(nums3) / sizeof(nums3[0]);
cout << "\nOriginal array: ";
for (int i = 0; i < arr_size; i++)
cout << nums3[i] << " ";
cout << "\nNumber of arithmetic slices: " << number_Of_Arithmetic_Slices(nums3, arr_size) << endl;
return 0;
}
Sample Output:
Original array: 1 2 3 9 4 5 6 Number of arithmetic slices: 2 Original array: 1 3 5 7 Number of arithmetic slices: 3 Original array: 2 1 3 4 7 Number of arithmetic slices: 0
Flowchart:
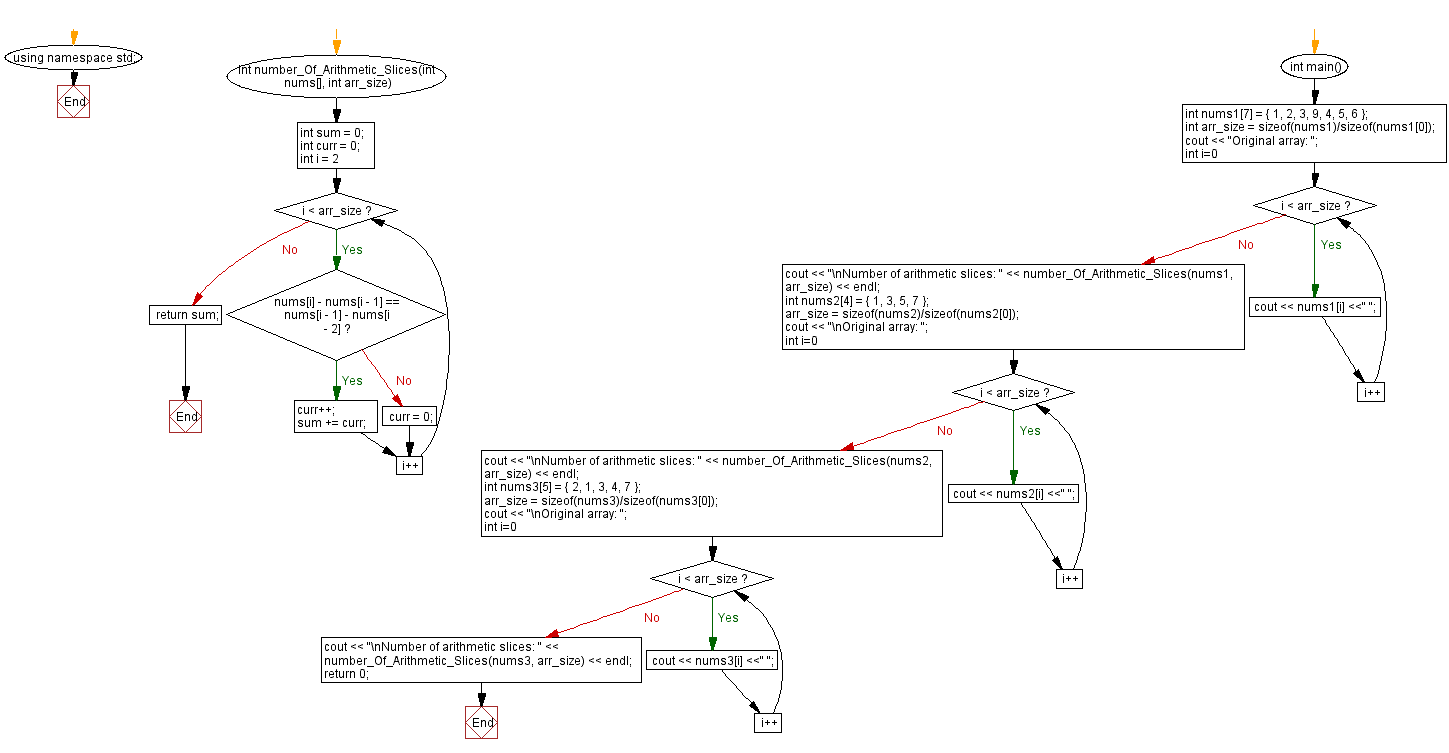
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Replace a given number until it become 1.
Next: Number as the multiplication of its prime factors.What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/math/cpp-math-exercise-32.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics