C++ Exercises: Number as the multiplication of its prime factors
33. Product of Prime Factors for Numbers 1 to n
Write a C++ program to count from 1 to a specified number and display each number as the product of its prime factors.
Test Data:
Display each number as the product of its prime factors:
From 1 to 23-
1: 1
2: 2
3: 3
4: 2 x 2
5: 5
6: 2 x 3
7: 7
8: 2 x 2 x 2
9: 3 x 3
10: 2 x 5
11: 11
12: 2 x 2 x 3
13: 13
14: 2 x 7
15: 3 x 5
16: 2 x 2 x 2 x 2
17: 17
18: 2 x 3 x 3
19: 19
20: 2 x 2 x 5
21: 3 x 7
22: 2 x 11
23: 23
Sample Solution:
C++ Code :
// Source: shorturl.at/bhjoP
#include <iostream>
#include <iomanip> // Library for manipulating output formatting
using namespace std;
// Function to calculate and display prime factors of a number
void getPrimeFactors(int li) {
int f = 2;
string res;
if (li == 1)
res = "1"; // For the number 1, there is only one factor which is 1 itself
else {
while (true) {
if (!(li % f)) { // If 'f' is a factor of 'li'
res += to_string(f); // Append 'f' to the result string
li /= f;
if (li == 1)
break; // If 'li' becomes 1, break the loop
res += " x "; // Add 'x' symbol to separate factors in the string
} else
f++; // Move to the next number to check if it's a factor
}
}
cout << res << "\n"; // Display the result string containing prime factors
}
int main(int argc, char* argv[]) {
int n = 23; // Define the upper limit to calculate prime factors (from 1 to 'n')
cout << "Display each number as the product of its prime factors:";
cout << "\nFrom 1 to " << n << "-\n";
for (int x = 1; x <= n; x++) {
cout << right << setw(4) << x << ": "; // Output formatting to align numbers
getPrimeFactors(x); // Call function to calculate and display prime factors
}
cout << endl;
return 0;
}
Sample Output:
Display each number as the product of its prime factors: From 1 to 23- 1: 1 2: 2 3: 3 4: 2 x 2 5: 5 6: 2 x 3 7: 7 8: 2 x 2 x 2 9: 3 x 3 10: 2 x 5 11: 11 12: 2 x 2 x 3 13: 13 14: 2 x 7 15: 3 x 5 16: 2 x 2 x 2 x 2 17: 17 18: 2 x 3 x 3 19: 19 20: 2 x 2 x 5 21: 3 x 7 22: 2 x 11 23: 23
Flowchart:
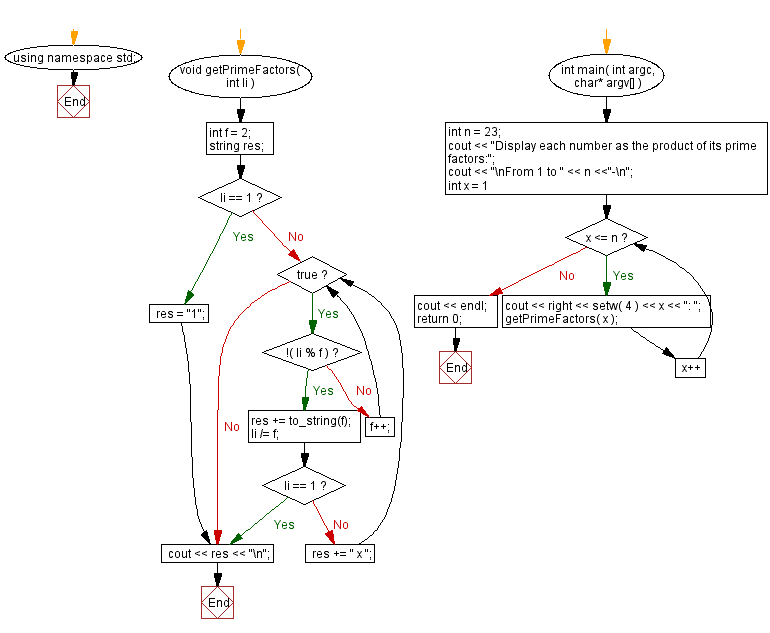
For more Practice: Solve these Related Problems:
- Write a C++ program to print numbers from 1 to n, each represented as the product of their prime factors using recursion.
- Write a C++ program that reads an integer n and outputs each number from 1 to n along with its prime factorization in formatted form.
- Write a C++ program to generate and display the prime decomposition for each number in a given range using trial division.
- Write a C++ program that iterates from 1 to n and prints each number as a multiplication of its prime factors using a helper function.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Number of arithmetic slices in an array of integers.
Next: Prime decomposition.What is the difficulty level of this exercise?