C++ Exercises: Get the fraction part from two given integers representing the numerator and denominator in string format
6. Get Fraction Part from Two Integers
Write a C++ program to get the fraction part from two given integers representing the numerator and denominator in string format.
Sample Input: x = 3
n = 2
Sample Output: 1.5
Sample Solution:
C++ Code :
#include <iostream>
#include <unordered_map>
using namespace std;
// Function to convert a fractional number to its decimal representation
string fraction_to_decimal(int numerator_part, int denominator_part) {
string result;
// Handling sign of the result
if ((numerator_part ^ denominator_part) >> 31 && numerator_part != 0) {
result = "-"; // If the signs are different, result is negative
}
// Taking absolute values for calculation
auto dvd_part = llabs(numerator_part);
auto dvs_part = llabs(denominator_part);
result += to_string(dvd_part / dvs_part); // Calculating integer part
dvd_part %= dvs_part;
if (dvd_part > 0) {
result += "."; // If there's a fractional part
}
unordered_map<long long, int> lookup; // Map to track remainder and position
// Loop to calculate the fractional part
while (dvd_part && !lookup.count(dvd_part)) {
lookup[dvd_part] = result.length(); // Storing the remainder's position
dvd_part *= 10;
result += to_string(dvd_part / dvs_part); // Append next decimal digit
dvd_part %= dvs_part; // Update the remainder
}
// If the remainder is repeated, it's a recurring decimal
if (lookup.count(dvd_part)) {
result.insert(lookup[dvd_part], "("); // Insert "(" at the repeating position
result.push_back(')'); // Add ")" at the end to indicate the recurring part
}
return result; // Return the decimal representation
}
int main(void) {
// Test cases for the fractional to decimal conversion
int x = 3;
int n = 2;
cout << "\nFractional part of " << x << " and " << n << " = " << fraction_to_decimal(x, n) << endl;
x = 4;
n = 7;
cout << "\nFractional part of " << x << " and " << n << " = " << fraction_to_decimal(x, n) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Fractional part of 3 and 2 = 1.5 Fractional part of 4 and 7 = 0.(571428)
Flowchart:
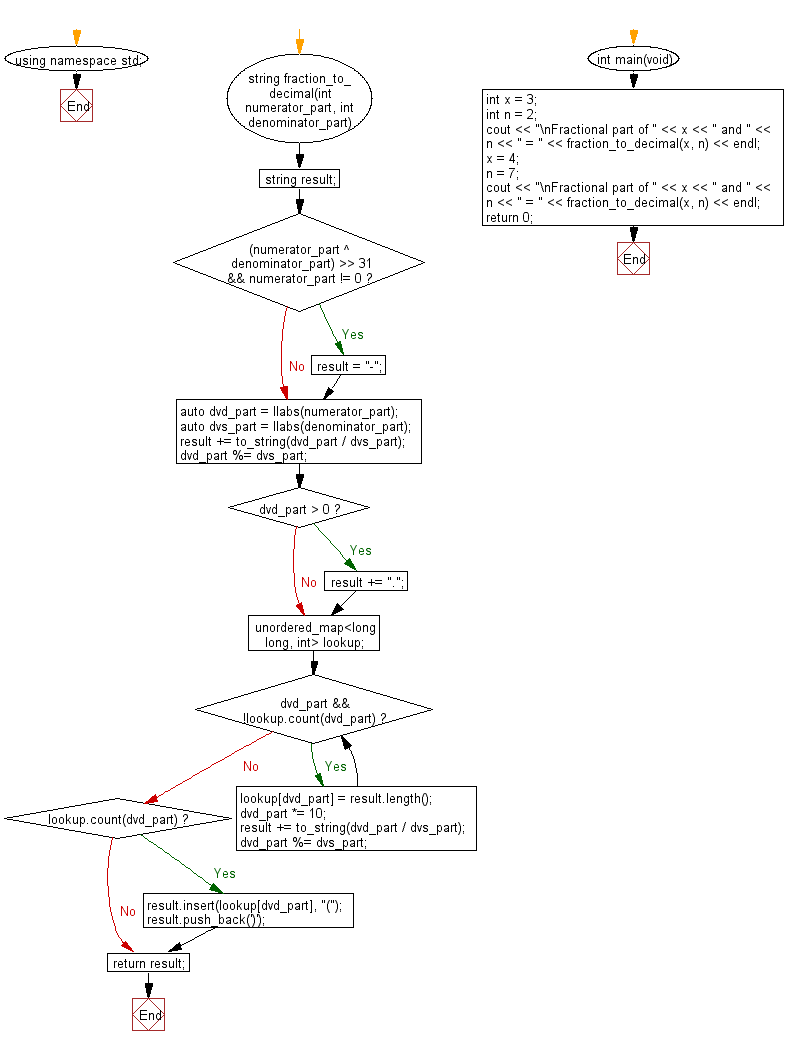
For more Practice: Solve these Related Problems:
- Write a C++ program that takes a numerator and denominator as input, computes the fractional part, and outputs the result as a floating-point number.
- Write a C++ program to read two integers representing a fraction and then convert it to a mixed number (integer part and fractional part).
- Write a C++ program to dynamically compute the division of two integers and return the precise decimal result using type casting.
- Write a C++ program that implements a function to divide two integers and output the result with a fixed number of decimal places.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to calculate x raised to the power n (xn).
Next: Write a C++ program to get the Excel column title that corresponds to a given column number (integer value).
What is the difficulty level of this exercise?