C++ Exercises: Get the Excel column title that corresponds to a given column number
7. Excel Column Title from Number
Write a C++ program to get the Excel column title that corresponds to a given column number (integer value).
For example:
1 -> A
2 -> B
3 -> C
...
26 -> Z
27 -> AA
28 -> AB
...
Sample Solution:
C++ Code :
#include <iostream>
using namespace std;
// Function to convert a number to Excel column title
string convert_num_to_Excel_Title(int num) {
if (num == 0) {
return ""; // If the number is 0, return an empty string
}
// Recursive call to convert the number to Excel title
return convert_num_to_Excel_Title((num - 1) / 26) + static_cast<char>((num - 1) % 26 + 'A');
}
int main(void) {
// Test cases to convert numbers to Excel column titles
int n = 2;
cout << "\nColumn Number n = " << n << " Excel column title = " << convert_num_to_Excel_Title(n) << endl;
n = 29;
cout << "\nColumn Number n = " << n << " Excel column title = " << convert_num_to_Excel_Title(n) << endl;
n = 153;
cout << "\nColumn Number n = " << n << " Excel column title = " << convert_num_to_Excel_Title(n) << endl;
return 0; // Return 0 to indicate successful completion
}
Sample Output:
Column Number n = 2 Excel column title = B Column Number n = 29 Excel column title = AC Column Number n = 153 Excel column title = EW
Flowchart:
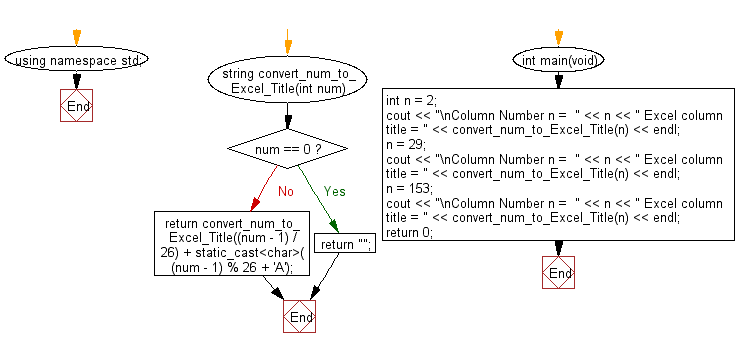
For more Practice: Solve these Related Problems:
- Write a C++ program to convert a given positive integer to its corresponding Excel column title using division and remainder operations.
- Write a C++ program that uses recursion to convert a column number into its Excel title by handling the base conversion iteratively.
- Write a C++ program to implement a function that converts numbers to Excel column titles and handles edge cases like 26, 27, and 52 correctly.
- Write a C++ program to generate Excel column titles for a range of numbers and print them in a formatted list.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to get the fraction part from two given integers representing the numerator and denominator in string format.
Next: Write a C++ program to get the column number (integer value) that corresponds to a column title as appear in an Excel sheet.
What is the difficulty level of this exercise?