C++ Exercises: Check whether a number is Lychrel number or not
11. Lychrel Number Check
Write a program in C++ to check whether a number is a Lychrel number or not.
Visual Presentation:
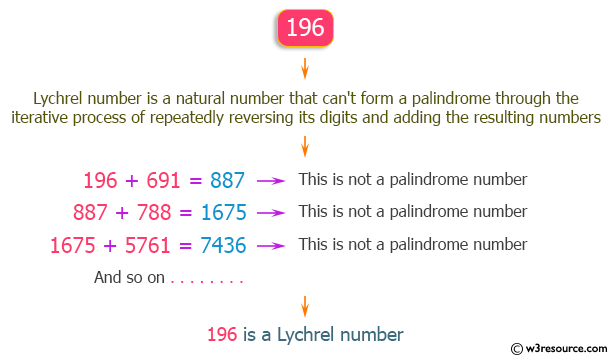
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Use the standard namespace
// Function to reverse a number
long long int numReverse(long long int number)
{
long long int rem = 0;
while (number > 0)
{
rem = (rem * 10) + (number % 10); // Extract digits in reverse order
number = number / 10; // Remove the last digit
}
return rem; // Return the reversed number
}
// Function to check if a number is a palindrome
bool is_Palindrome(long long int num)
{
return (num == numReverse(num)); // Check if the number is equal to its reverse
}
// Function to check if a number is a Lychrel number
bool isLychrel(int num, const int iterCount = 500)
{
long long int temp = num;
long long int rev;
for (int i = 0; i < iterCount; i++)
{
rev = numReverse(temp); // Get the reversed number
if (is_Palindrome(rev + temp)) // Check if the sum of the number and its reverse is a palindrome
return false; // If it's a palindrome, it's not a Lychrel number
temp = temp + rev; // Update temp with the sum
}
return true; // If after iterations no palindrome is found, it's a Lychrel number
}
int main()
{
int lyno;
bool l;
cout << "\n\n Check whether a given number is a Lychrel number: \n"; // Display a message for user
cout << " ------------------------------------------------------\n";
cout << " Input a number: ";
cin >> lyno; // Input a number from the user
l = isLychrel(lyno); // Check if the input number is a Lychrel number
if (l == false)
{
cout << " The number is not a Lychrel number." << endl; // Display result if it's not a Lychrel number
}
else
{
cout << " The number is a Lychrel number." << endl; // Display result if it's a Lychrel number
}
return 0; // End of the program
}
Sample Output:
Check whether a given number is a Lychrel number: ------------------------------------------------------ Input a number: 196 The number is a Lychrel number.
Flowchart:
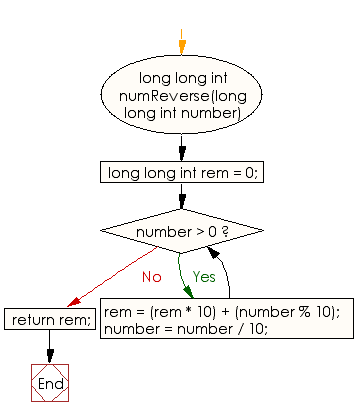
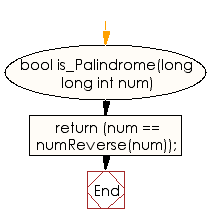
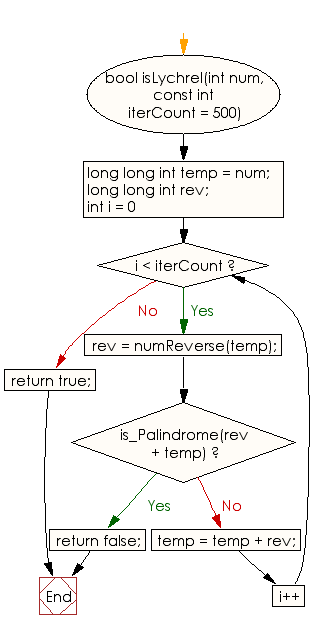
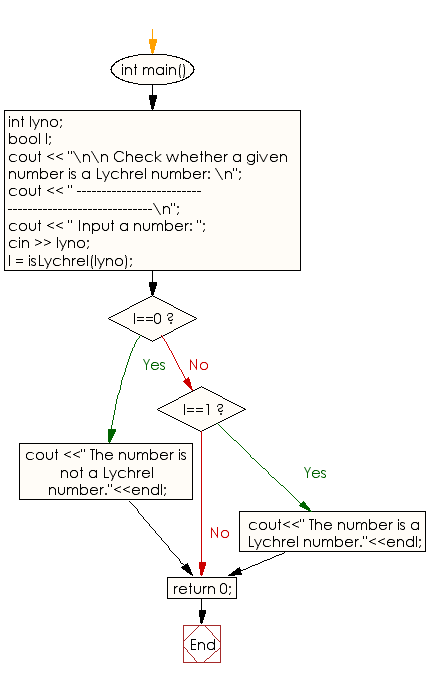
For more Practice: Solve these Related Problems:
- Write a C++ program to determine if a number is a Lychrel candidate by iterating digit reversals with a limit.
- Write a C++ program to check for Lychrel numbers using string reversal and loop counters.
- Write a C++ program to test if a number is Lychrel by comparing iterative sums to the original number.
- Write a C++ program to verify Lychrel numbers using recursion with a set maximum number of iterations.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to generate and show all Kaprekar numbers less than 1000.
Next: Write a program in C++ to find the Lychrel numbers and the number of Lychrel number within the range 1 to 1000 (after 500 iteration).
What is the difficulty level of this exercise?