C++ Exercises: Find the Lychrel numbers and the number of Lychrel number within the range 1 to 1000
12. Find Lychrel Numbers Between 1 and 1000 (After 500 Iterations)
Write a C++ program to find the Lychrel numbers and the number of Lychrel numbers in the range 1 to 1000 (after 500 iterations).
Visual Presentation:
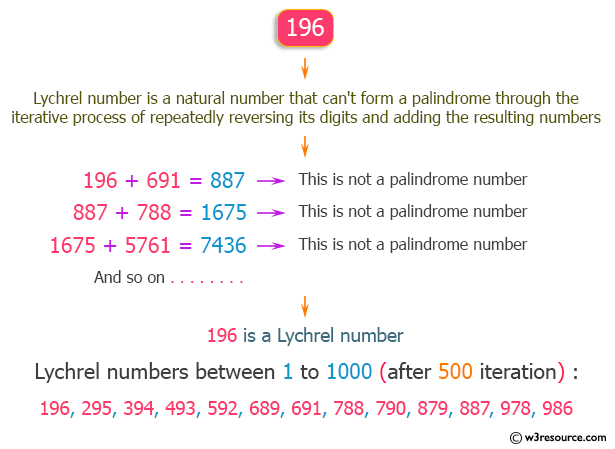
Sample Solution:
C++ Code :
#include <iostream> // Include the input/output stream library
using namespace std; // Use the standard namespace
// Function to reverse a number
long long int numReverse(long long int number)
{
long long int rem = 0;
while (number > 0)
{
rem = (rem * 10) + (number % 10); // Extract digits in reverse order
number = number / 10; // Remove the last digit
}
return rem; // Return the reversed number
}
// Function to check if a number is a palindrome
bool is_Palindrome(long long int num)
{
return (num == numReverse(num)); // Check if the number is equal to its reverse
}
// Function to check if a number is a Lychrel number
bool isLychrel(int num, const int iterCount = 500)
{
long long int temp = num;
long long int rev;
for (int i = 0; i < iterCount; i++)
{
rev = numReverse(temp); // Get the reversed number
if (is_Palindrome(rev + temp)) // Check if the sum of the number and its reverse is a palindrome
return false; // If it's a palindrome, it's not a Lychrel number
temp = temp + rev; // Update temp with the sum
}
return true; // If after iterations no palindrome is found, it's a Lychrel number
}
int main()
{
int lyno, ctr = 0, i; // Initialize variables for number, counter, and iteration
bool l; // Initialize a boolean variable to check Lychrel property
cout << "\n\n Find the Lychrel numbers between 1 to 1000(after 500 iteration): \n"; // Display a message for user
cout << " ----------------------------------------------------------------------\n";
cout << " The Lychrel numbers are : ";
for (i = 1; i <= 1000; i++) // Loop through numbers from 1 to 1000
{
lyno = i; // Assign the current number
l = isLychrel(lyno); // Check if the number is a Lychrel number
if (l == true) // If it's a Lychrel number
{
ctr++; // Increment the counter
cout << lyno << " "; // Display the Lychrel number
}
}
cout << endl;
cout << " The number of Lychrel numbers are: " << ctr << endl; // Display the total count of Lychrel numbers
return 0; // End of the program
}
Sample Output:
Find the Lychrel numbers between 1 to 1000(after 500 iteration): ---------------------------------------------------------------------- The Lychrel numbers are : 196 295 394 493 592 689 691 788 790 879 887 978 986 The number of Lychrel numbers are: 13
Flowchart:
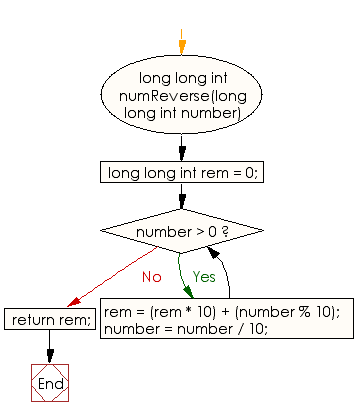
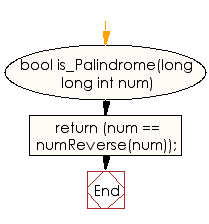
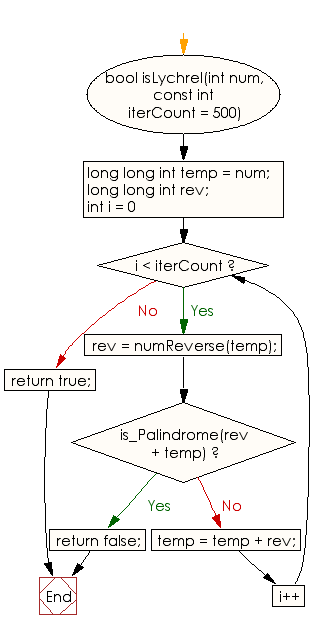
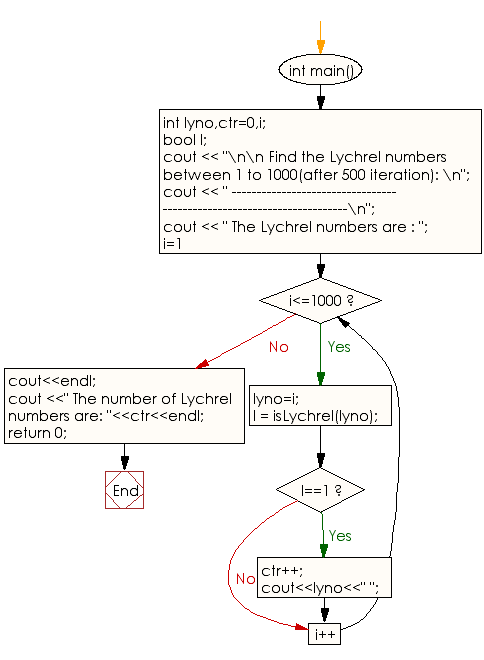
For more Practice: Solve these Related Problems:
- Write a C++ program to list all Lychrel numbers in a range by applying 500 iterations for each candidate.
- Write a C++ program to identify Lychrel numbers between 1 and 1000 using optimized string manipulation.
- Write a C++ program to generate Lychrel numbers within a range by integrating iterative functions and counters.
- Write a C++ program to check for Lychrel numbers using multi-threading to process candidates concurrently.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a number is Lychrel number or not.
Next: Write a program in C++ to generate and show the first 15 Narcissistic decimal numbers.
What is the difficulty level of this exercise?