C++ Exercises: Check a number is a Happy or not
C++ Numbers: Exercise-16 with Solution
Write a program in C++ to determine whether a number is Happy or not.
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include the necessary libraries
using namespace std; // Use the standard namespace
// Function to calculate the sum of squares of digits of a number
int SumOfSquNum(int givno)
{
int SumOfSqr = 0; // Initialize sum of squares as 0
while (givno)
{
// Calculate sum of squares by squaring each digit and summing them up
SumOfSqr += (givno % 10) * (givno % 10);
givno /= 10; // Move to the next digit
}
return SumOfSqr; // Return the calculated sum of squares
}
// Function to check if a number is a Happy number
bool checkHappy(int chkhn)
{
int slno, fstno; // Declare variables to keep track of sequence
slno = fstno = chkhn; // Initialize both variables with the given number
do
{
// One pointer moves by calculating sum of squares of digits once
slno = SumOfSquNum(slno);
// Another pointer moves by calculating sum of squares of digits twice
fstno = SumOfSquNum(SumOfSquNum(fstno));
}
// Continue until both pointers meet or they reach the Happy number condition (sum equals 1)
while (slno != fstno);
return (slno == 1); // Return true if the number is a Happy number, else return false
}
// Main function
int main()
{
int hyno; // Variable to store the user input number
cout << "\n\n Check whether a number is a Happy number or not: \n"; // Display message about the program
cout << " ---------------------------------------------------\n";
cout << " Input a number: "; // Prompt the user to enter a number
cin >> hyno; // Read the user input
// Check if the input number is a Happy number and display the result
if (checkHappy(hyno))
cout << hyno << " is a Happy number\n";
else
cout << hyno << " is not a Happy number\n";
return 0; // Return 0 to signify successful completion of the program
}
Sample Output:
Check whether a number is Happy number or not: --------------------------------------------------- Input a number: 23 23 is a Happy number
Flowchart:
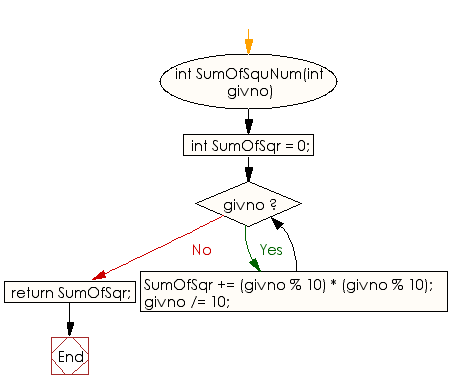
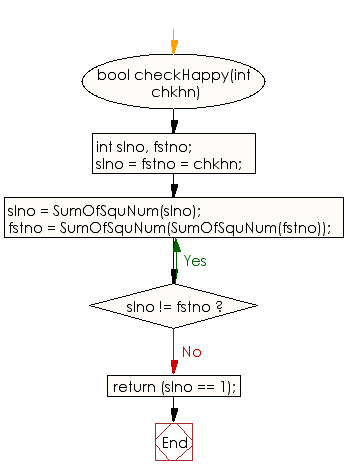
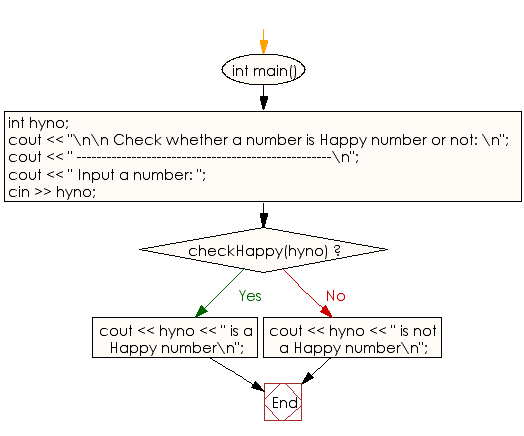
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to display the first 10 Catlan numbers.
Next: Write a program in C++ to find the Happy numbers between 1 to 1000.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/numbers/cpp-numbers-exercise-16.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics