C++ Exercises: Find the Happy numbers between 1 to 1000
17. Find Happy Numbers Between 1 and 1000
Write a C++ program to find the Happy numbers between 1 and 1000.
Sample Solution:
C++ Code :
#include <bits/stdc++.h> // Include the necessary libraries
using namespace std; // Use the standard namespace
// Function to calculate the sum of squares of digits of a number
int SumOfSquNum(int givno)
{
int SumOfSqr = 0; // Initialize sum of squares as 0
while (givno)
{
// Calculate sum of squares by squaring each digit and summing them up
SumOfSqr += (givno % 10) * (givno % 10);
givno /= 10; // Move to the next digit
}
return SumOfSqr; // Return the calculated sum of squares
}
// Function to check if a number is a Happy number
bool checkHappy(int chkhn)
{
int slno, fstno; // Declare variables to keep track of sequence
slno = fstno = chkhn; // Initialize both variables with the given number
do
{
// One pointer moves by calculating sum of squares of digits once
slno = SumOfSquNum(slno);
// Another pointer moves by calculating sum of squares of digits twice
fstno = SumOfSquNum(SumOfSquNum(fstno));
}
// Continue until both pointers meet or they reach the Happy number condition (sum equals 1)
while (slno != fstno);
return (slno == 1); // Return true if the number is a Happy number, else return false
}
// Main function
int main()
{
int j, ctr; // Declare variables to iterate and count
cout << "\n\n Find the Happy numbers between 1 to 1000: \n"; // Display message about the program
cout << " ----------------------------------------------\n";
cout << " The Happy numbers between 1 to 1000 are: "<<endl;
// Loop through numbers from 1 to 1000 to find and display Happy numbers
for (j = 1; j <= 1000; j++)
{
// Check if the current number is a Happy number and display it
if (checkHappy(j))
cout << j << " ";
}
cout << endl; // Move to the next line after displaying the Happy numbers
}
Sample Output:
Find the Happy numbers between 1 to 1000: ---------------------------------------------- The Happy numbers between 1 to 1000 are: 1 7 10 13 19 23 28 31 32 44 49 68 70 79 82 86 91 94 97 100 103 109 129 130 133 139 167 176 188 190 19 2 193 203 208 219 226 230 236 239 262 263 280 291 293 301 302 310 313 319 320 326 329 331 338 356 362 365 367 368 376 379 383 386 391 392 397 404 409 440 446 464 469 478 487 490 496 536 556 563 565 566 608 617 622 623 632 635 637 638 644 649 653 655 656 665 671 673 680 683 694 700 709 716 736 739 748 7 61 763 784 790 793 802 806 818 820 833 836 847 860 863 874 881 888 899 901 904 907 910 912 913 921 92 3 931 932 937 940 946 964 970 973 989 998 1000
Flowchart:
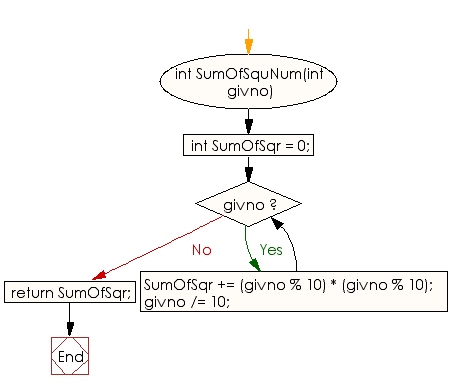
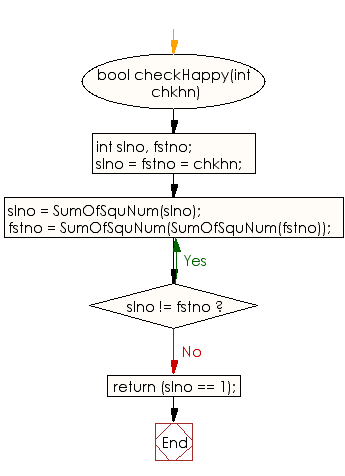
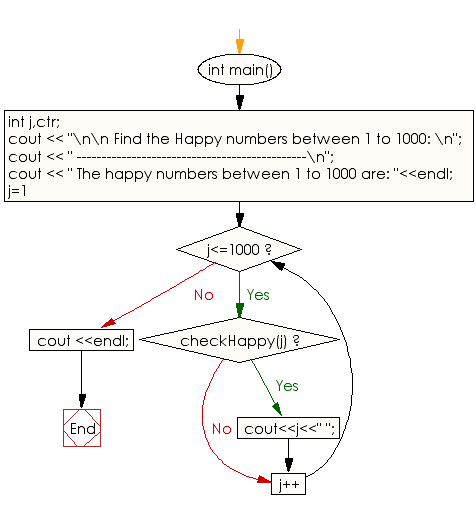
For more Practice: Solve these Related Problems:
- Write a C++ program to list all happy numbers between 1 and 1000 using an optimized digit-square summing function.
- Write a C++ program to generate happy numbers within a range by leveraging parallel processing for large ranges.
- Write a C++ program to compute and display happy numbers between 1 and 1000 with formatted output.
- Write a C++ program to identify happy numbers in a range by combining recursion with cycle detection.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check a number is a Happy or not.
Next: Write a program in C++ to check whether a number is Disarium or not.
What is the difficulty level of this exercise?