C++ Exercises: Find Disarium numbers between 1 to 1000
C++ Numbers: Exercise-19 with Solution
Write a C++ program to find Disarium numbers between 1 and 1000.
Visual Presentation:
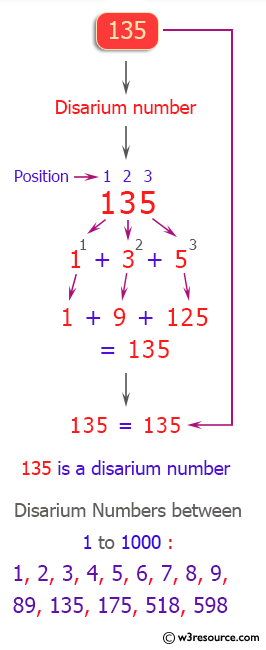
Sample Solution:
C++ Code :
#include<bits/stdc++.h> // Include necessary libraries
using namespace std; // Use the standard namespace
// Function to count the number of digits in a number
int DigiCount(int n)
{
int ctr_digi = 0; // Counter for digits initialized to 0
int tmpx = n; // Temporary variable to store the number
// Loop to count the digits by dividing the number by 10 until it becomes zero
while (tmpx)
{
tmpx = tmpx / 10; // Divide the number by 10
ctr_digi++; // Increment digit counter
}
return ctr_digi; // Return the count of digits in the number
}
// Function to check if a number is a Disarium Number
bool chkDisarum(int n)
{
int ctr_digi = DigiCount(n); // Calculate the count of digits in the number
int s = 0; // Initialize sum of powered digits to 0
int x = n; // Temporary variable to store the number
int pr; // Variable to store digits extracted from the number
// Loop to calculate the sum of powered digits
while (x)
{
pr = x % 10; // Extract the last digit of the number
s = s + pow(pr, ctr_digi--); // Add the powered digit to the sum
x = x / 10; // Remove the last digit from the number
}
return (s == n); // Return true if the sum of powered digits is equal to the original number
}
// Main function
int main()
{
int i; // Loop variable
cout << "\n\n Find Disarium Numbers between 1 to 1000: \n"; // Display message about the program
cout << " ---------------------------------------------\n";
cout << " The Disarium numbers are: "<<endl; // Display header for Disarium numbers
// Loop through numbers from 1 to 1000 to find and display Disarium numbers
for(i = 1; i <= 1000; i++)
{
if (chkDisarum(i)) // Check if the number is a Disarium number
cout << i << " "; // Display Disarium number if found
}
cout << endl; // Print newline after displaying all Disarium numbers
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Find Disarium Numbers between 1 to 1000: --------------------------------------------- The Disarium numbers are: 1 2 3 4 5 6 7 8 9 89 135 175 518 598
Flowchart:
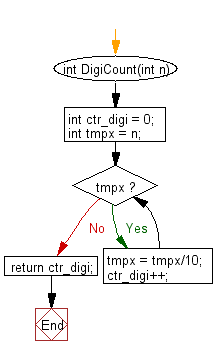
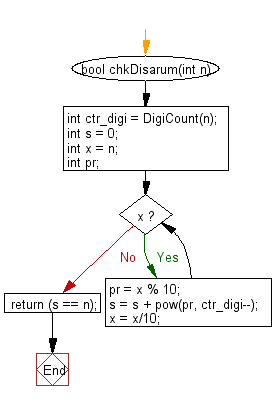
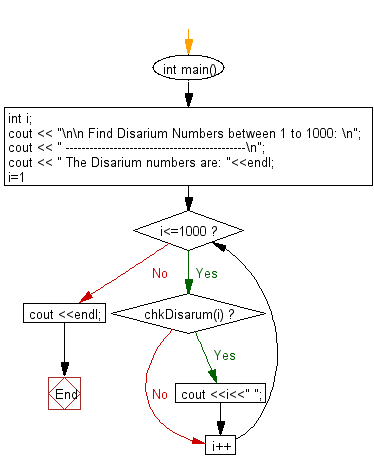
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to check whether a number is Disarium or not.
Next: Write a program in C++ to check if a number is Harshad Number or not.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/numbers/cpp-numbers-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics