C++ Exercises: Check whether a given number is palindrome or not
38. Palindrome Number Check
Write a C++ program to check whether a given number is a palindrome or not.
Sample Solution:
C++ Code :
#include <iostream> // Including the input-output stream library
using namespace std; // Using the standard namespace
int main() // Main function
{
int i, n1, r, s = 0; // Declare integer variables: i for iteration, n1 for input number, r for remainder, s for storing reversed number
cout << "\n\n Check whether a given number is palindrome or not: \n"; // Display purpose
cout << " -------------------------------------------------------\n"; // Display purpose
cout << " Input a number: "; // Prompt user for input
cin >> n1; // Input the number
for (i = n1; i > 0; ) // Loop to reverse the number
{
r = i % 10; // Extract the last digit of the number
s = s * 10 + r; // Build the reversed number by adding the extracted digit
i = i / 10; // Remove the last digit from the number
}
if (s == n1) // Check if the reversed number is equal to the original number
{
cout << " " << n1 << " is a Palindrome Number." << endl; // If equal, display that it's a palindrome
}
else
{
cout << " " << n1 << " is not a Palindrome Number." << endl; // If not equal, display that it's not a palindrome
}
}
Sample Output:
Check whether a given number is palindrome or not: ------------------------------------------------------- Input a number: 141 141 is a Palindrome Number.
Flowchart:
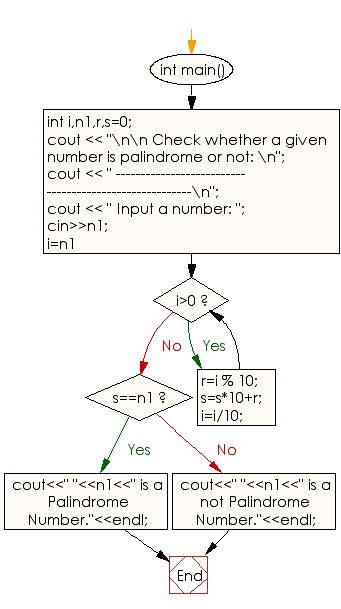
For more Practice: Solve these Related Problems:
- Write a C++ program to check if a number is a palindrome by reversing its digits using a while-loop.
- Write a C++ program to verify palindrome numbers by converting the number to a string and comparing reversed strings.
- Write a C++ program to determine palindromicity using recursion to reverse the number digit by digit.
- Write a C++ program to test for palindrome numbers with error handling for negative inputs.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C++ to find Narcissistic decimal numbers within a specific range.
Next: Write a program in C++ to print the first 20 numbers of the Pell series.
What is the difficulty level of this exercise?