C++ Recursion: Counting occurrences of an element in an array with recursive function
C++ Recursion: Exercise-11 with Solution
Write a C++ program to implement a recursive function to count the number of occurrences of a specific element in an array of integers.
Sample Solution:
C Code:
// Recursive function to count the number of occurrences of a specific element in an array
#include <iostream>
// Function to count occurrences of a specific element in an array
int countOccurrences(int nums[], int size, int element) {
// Base case: if the array is empty, return 0
if (size == 0)
return 0;
// Recursive case: if the first element matches the target element,
// increment the count and recursively search in the rest of the array
if (nums[0] == element)
return 1 + countOccurrences(nums + 1, size - 1, element);
else
return countOccurrences(nums + 1, size - 1, element);
}
int main() {
int nums[] = {3, 4, 5, 7, 3, 9, 5, 3, 5, 9, 3, 4, 3, 5};
int size = sizeof(nums) / sizeof(nums[0]);
std::cout << "Array elements: " << std::endl;
// loop through the array elements
for (size_t i = 0; i < size; i++) {
std::cout << nums[i] << ' ';
}
int element;
std::cout << "\nCount the number of occurrences of a specific element in the said array:";
std::cout << "\nInput an element: ";
std::cin >> element;
// Count the occurrences of the element using recursion
int count = countOccurrences(nums, size, element);
std::cout << "Number of occurrences of " << element << ": " << count << std::endl;
return 0;
}
Sample Output:
Array elements: 3 4 5 7 3 9 5 3 5 9 3 4 3 5 Count the number of occurrences of a specific element in the said array: Input an element: 7 Number of occurrences of 7: 1
3 4 5 7 3 9 5 3 5 9 3 4 3 5 Count the number of occurrences of a specific element in the said array: Input an element: 3 Number of occurrences of 3: 5
Explanation:
In the above exercise,
- The "countOccurrences()" function takes an array (nums), the array size (size), and the target element (element) as parameters.
- It uses recursion to count the number of occurrences of the target element in the array.
- The base case is when the array size becomes 0, in which case the function returns 0.
- In the recursive case, the function checks if the first element of the array matches the target element.
- If it does, the function increments the count by 1 and recursively calls itself with the rest of the array (nums + 1) and the updated size (size - 1).
- If the first element doesn't match the target element, the function recursively calls itself with the rest of the array and the updated size, without incrementing the count.
- The function returns the final count of occurrences.
- The "main()" function initializes an array of integers, accepts the user to enter the element to count its occurrences.
- It calls the "countOccurrences()" function to count the occurrences of the element, and then displays the result.
Flowchart:
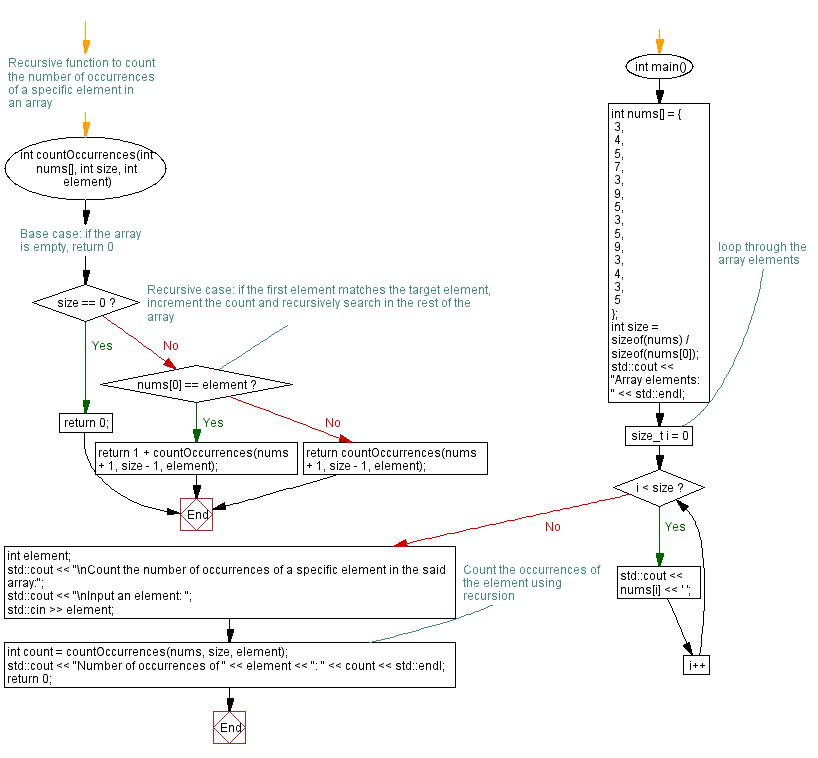
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Greatest common divisor (GCD) with recursive function.
Next C++ Exercise: Generating all permutations of a string with recursive function.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics