C++ Bubble sort algorithm Exercise: Sort an array of elements using the Bubble sort algorithm
C++ Sorting: Exercise-4 with Solution
Write a C++ program to sort an array of elements using the Bubble sort algorithm.
Sample Solution:
C++ Code :
// Including necessary C++ libraries
#include <algorithm>
#include <iostream>
#include <iterator>
// Function to perform bubble sort algorithm
template <typename RandomAccessIterator>
void bubble_sort(RandomAccessIterator begin, RandomAccessIterator end) {
// Flag to indicate if elements were swapped during iteration
bool swapped = true;
// Loop until the range [begin, end) is sorted or end is reached
while (begin != end-- && swapped) {
swapped = false; // Resetting swapped flag for each iteration
// Iterating through the range using the bubble sort algorithm
for (auto i = begin; i != end; ++i) {
if (*(i + 1) < *i) { // Comparing adjacent elements and swapping if necessary
std::iter_swap(i, i + 1);
swapped = true; // Setting the flag to indicate swapping occurred
}
}
}
}
// Main function
int main() {
// Initializing an array of integers for sorting
int a[] = {125, 0, 695, 3, -256, -5, 214, 44, 55};
// Displaying the original numbers in the array
std::cout << "Original numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Sorting the array using bubble_sort function
bubble_sort(std::begin(a), std::end(a));
// Displaying the sorted array after bubble sort
std::cout << "Sorted array:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Returning 0 to indicate successful execution
return 0;
}
Sample Output:
Original numbers: 125 0 695 3 -256 -5 214 44 55 Sorted array: -256 -5 0 3 44 55 125 214 695
Flowchart:
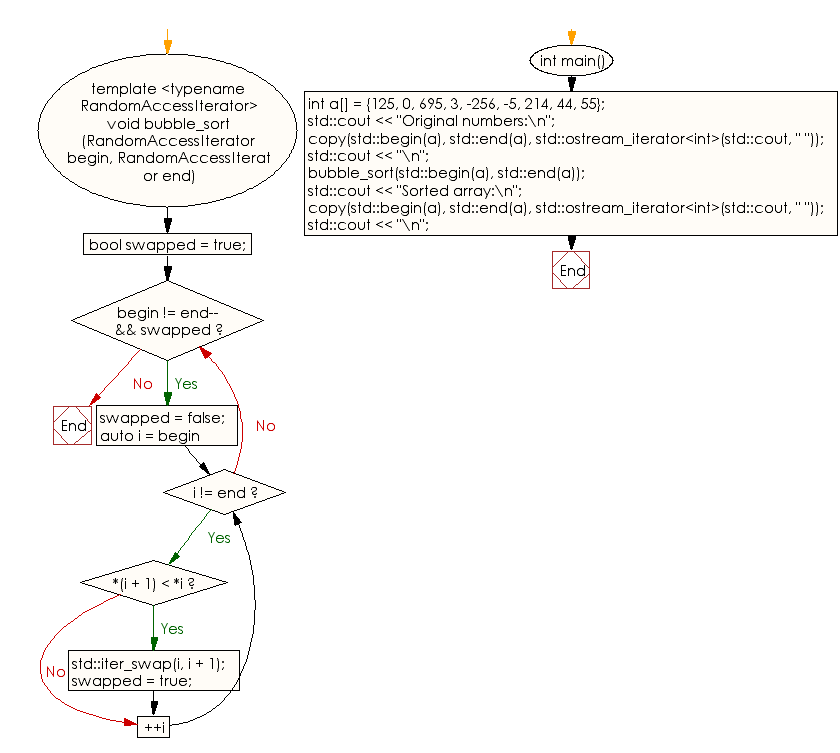
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort a list of numbers using Bogosort algorithm.
Next: Write a C++ program to sort an array of elements using the Cocktail sort algorithm.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/sorting-and-searching/cpp-sorting-and-searching-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics