C++ Cocktail sort algorithm Exercise: Sort an array of elements using the Cocktail sort algorithm
C++ Sorting: Exercise-5 with Solution
Write a C++ program to sort an array of elements using the Cocktail sort algorithm.
Sample Solution:
C++ Code :
// Including necessary C++ libraries
#include <algorithm>
#include <iostream>
#include <iterator>
// Function to perform cocktail shaker sort algorithm
template <typename RandomAccessIterator>
void cocktail_sort(RandomAccessIterator begin, RandomAccessIterator end) {
bool swapped = true;
// Iterate until no more swaps are made or the range is sorted
while (begin != end-- && swapped) {
swapped = false;
// Forward pass: Move the larger elements to the end
for (auto i = begin; i != end; ++i) {
if (*(i + 1) < *i) {
std::iter_swap(i, i + 1);
swapped = true;
}
}
// If no elements were swapped, the list is sorted, break the loop
if (!swapped) {
break;
}
swapped = false;
// Backward pass: Move the smaller elements to the beginning
for (auto i = end - 1; i != begin; --i) {
if (*i < *(i - 1)) {
std::iter_swap(i, i - 1);
swapped = true;
}
}
++begin; // Move the starting point ahead after each iteration
}
}
// Main function
int main() {
// Initializing an array of integers for sorting
int a[] = {100, 2, 56, 200, -52, 3, 99, 33, 177, -199};
// Sorting the array using cocktail_sort function
cocktail_sort(std::begin(a), std::end(a));
// Displaying the sorted array after cocktail shaker sort
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Returning 0 to indicate successful execution
return 0;
}
Sample Output:
Original numbers: 100, 2, 56, 200, -52, 3, 99, 33, 177, -199 Sorted numbers: -199 -52 2 3 33 56 99 100 177 200
Flowchart:
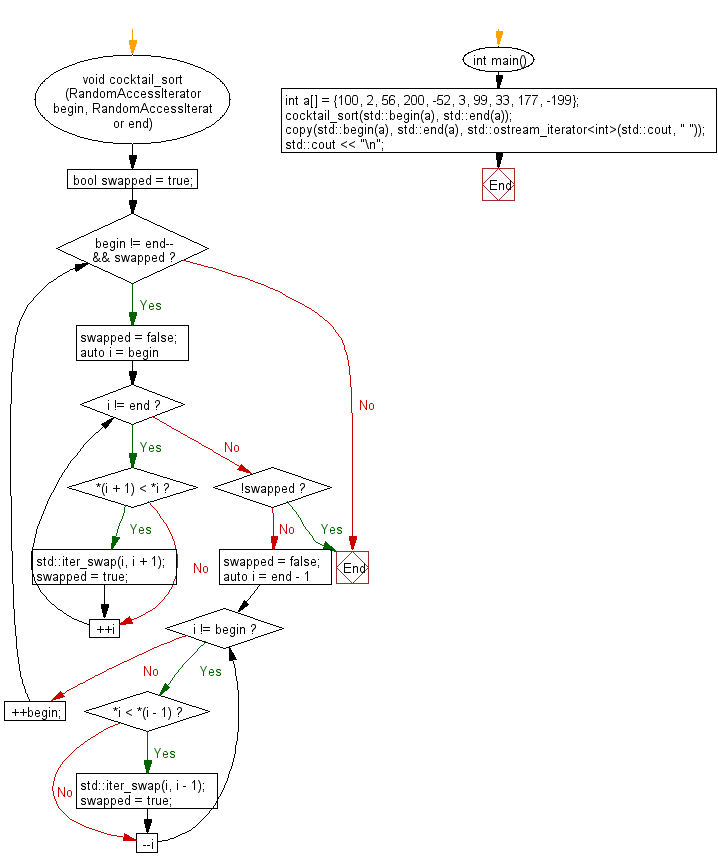
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort an array of elements using the Bubble sort algorithm.
Next: Write a C++ program to sort an array of elements using the Counting sort algorithm.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics