C++ Counting sort algorithm Exercise: Sort an array of elements using the Counting sort algorithm
6. Sort an Array Using the Counting Sort Algorithm
Write a C++ program to sort an array of elements using the Counting sort algorithm.
Sample Solution:
C++ Code :
// Including necessary C++ libraries
#include <algorithm>
#include <iterator>
#include <iostream>
#include <vector>
// Function to perform counting sort algorithm
template<typename ForwardIterator>
void counting_sort(ForwardIterator begin, ForwardIterator end) {
// Finding the minimum and maximum elements in the range
auto min_max = std::minmax_element(begin, end);
// If the range is empty, return as there's no sorting needed
if (min_max.first == min_max.second) {
return;
}
// Determining the minimum and maximum values
auto min = *min_max.first;
auto max = *min_max.second;
// Creating a count vector to store the frequency of elements
std::vector<unsigned> count((max - min) + 1, 0u);
// Counting the occurrences of each element in the range
for (auto i = begin; i != end; ++i) {
++count[*i - min];
}
// Reconstructing the sorted sequence using count information
for (auto i = min; i <= max; ++i) {
for (auto j = 0; j < count[i - min]; ++j) {
*begin++ = i;
}
}
}
// Main function
int main() {
// Initializing an array of integers for sorting
int a[] = {125, 0, 695, 3, -256, -5, 214, 44, 55};
// Displaying the original numbers in the array
std::cout << "Original numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Sorting the array using counting_sort function
counting_sort(std::begin(a), std::end(a));
// Displaying the sorted numbers after counting sort
std::cout << "Sorted numbers:\n";
std::copy(std::begin(a), std::end(a), std::ostream_iterator<int>(std::cout, " "));
std::cout << "\n";
// Returning 0 to indicate successful execution
return 0;
}
Sample Output:
Original numbers: 125 0 695 3 -256 -5 214 44 55 Sorted numbers: -256 -5 0 3 44 55 125 214 695
Flowchart:
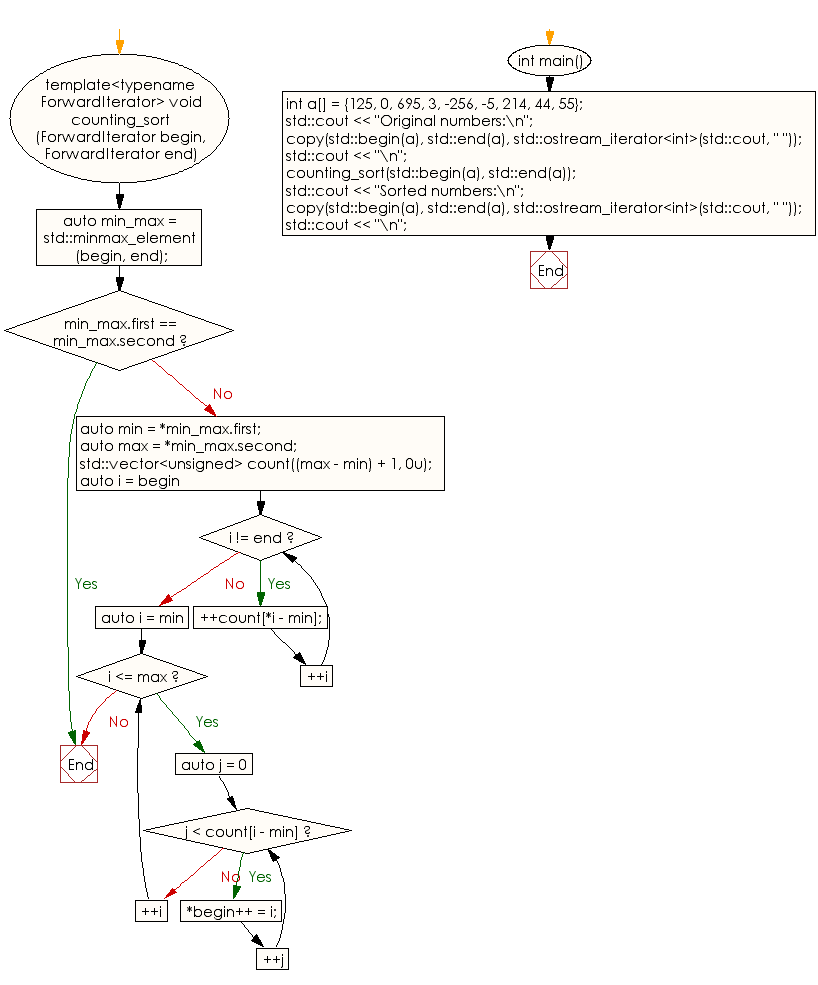
For more Practice: Solve these Related Problems:
- Write a C++ program to implement counting sort on an array that includes negative integers by applying an offset.
- Write a C++ program to display the frequency array generated during counting sort before constructing the output.
- Write a C++ program to implement counting sort using vectors and iterators for dynamic range inputs.
- Write a C++ program to perform counting sort and measure its execution time on large datasets.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to sort an array of elements using the Cocktail sort algorithm.
Next: Write a C++ program to sort an array of elements using the Gnome sort algorithm.
What is the difficulty level of this exercise?