C++ Stack Exercises: Sort the elements of a stack (using a linked list)
19. Sort the Elements of a Linked List-Based Stack
Write a C++ program to sort the elements of a stack (using a linked list).
Test Data:
Input some elements onto the stack:
Stack elements are: 0 1 3 5 6
Sorted elements of the said stack:
Stack elements are: 6 5 3 1 0
Sample Solution:
C++ Code:
#include <iostream>
using namespace std;
// Define the node structure for the linked list
struct Node {
int data;
Node* next;
};
class Stack {
private:
// This variable keeps track of the stack size
int size;
public:
// Top-of-stack pointer
Node* top;
// Constructor to initialize an empty stack
Stack() {
top = NULL; // Initialize top pointer to NULL for an empty stack
size = 0; // Initialize the size of the stack to zero
}
// Function to push an element onto the stack
void push(int x) {
Node* new_Node = new Node();
new_Node->data = x;
new_Node->next = top;
top = new_Node;
size++;
}
// Function to push elements onto the stack (helper function)
void push_stk(Node** topRef, int data) {
Node* newNode = new Node(); // Create a new node
newNode->data = data; // Assign data to the new node
newNode->next = *topRef; // Set the next of the new node to the current top node
*topRef = newNode; // Update the top reference to the new node
}
// Function to pop an element from the stack
void pop() {
if (top == NULL) {
cout << "Stack is empty!" << endl; // Display message if the stack is empty
return;
}
Node* temp = top; // Store the current top node
top = top->next; // Move top to the next node
size--; // Decrement the size of the stack
delete temp; // Delete the previous top node
}
// Function to sort the elements of the stack in ascending order
void sortStack(Node** top) {
if (*top == NULL || (*top)->next == NULL) {
// There is only one element in the stack, or the stack is empty, so it is already sorted.
return;
}
Node* prev = NULL;
Node* current = *top;
Node* next = NULL;
// Reverse the stack
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
*top = prev; // Update the top pointer with the reversed stack
// Temporary stack to sort elements
Node* tempStack = NULL;
while (*top != NULL) {
int temp = (*top)->data;
(*top) = (*top)->next;
// Move elements to the temporary stack to sort
while (tempStack != NULL && tempStack->data > temp) {
push_stk(top, tempStack->data);
tempStack = tempStack->next;
}
push_stk(&tempStack, temp); // Push the current element into the temporary stack
}
// Set the original stack to the elements that were sorted.
*top = tempStack;
}
// Function to display the elements of the stack
void display() {
if (top == NULL) {
cout << "Stack is empty!" << endl; // Display message if the stack is empty
return;
}
Node* temp = top; // Create a temporary node to traverse the stack
cout << "Stack elements are: ";
while (temp != NULL) {
cout << temp->data << " "; // Display the data of each node
temp = temp->next; // Move to the next node
}
cout << endl;
}
};
int main() {
Stack stk; // Create an object of Stack class
cout << "Input some elements onto the stack:\n";
stk.push(6);
stk.push(5);
stk.push(3);
stk.push(1);
stk.push(0);
stk.display(); // Display the elements in the stack
cout << "\nSorted elements of the said stack:\n";
stk.sortStack(&stk.top); // Sort the stack elements in ascending order
stk.display(); // Display the sorted stack elements
cout << "\nInput two more elements:\n";
stk.push(35);
stk.push(29);
stk.display(); // Display the updated elements in the stack
cout << "\nSorted elements of the said stack:\n";
stk.sortStack(&stk.top); // Sort the stack elements in ascending order again
stk.display(); // Display the sorted stack elements
return 0;
}
Sample Output:
Input some elements onto the stack: Stack elements are: 0 1 3 5 6 Sorted elements of the said stack: Stack elements are: 6 5 3 1 0 Input two more elements: Stack elements are: 29 35 6 5 3 1 0 Sorted elements of the said stack: Stack elements are: 35 29 6 5 3 1 0
Flowchart:
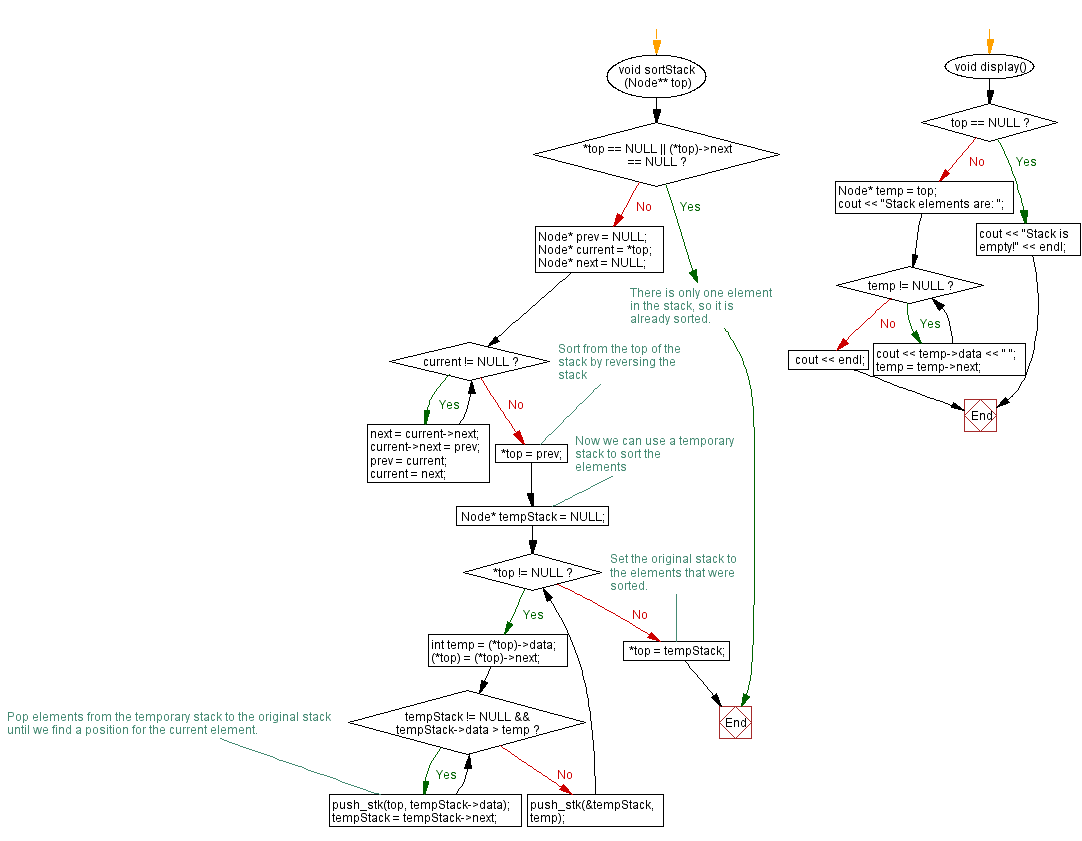
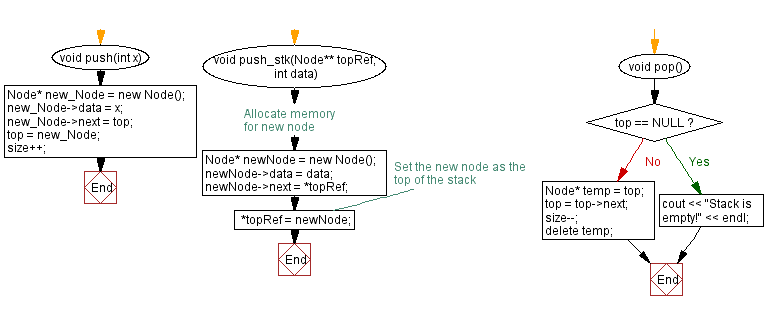
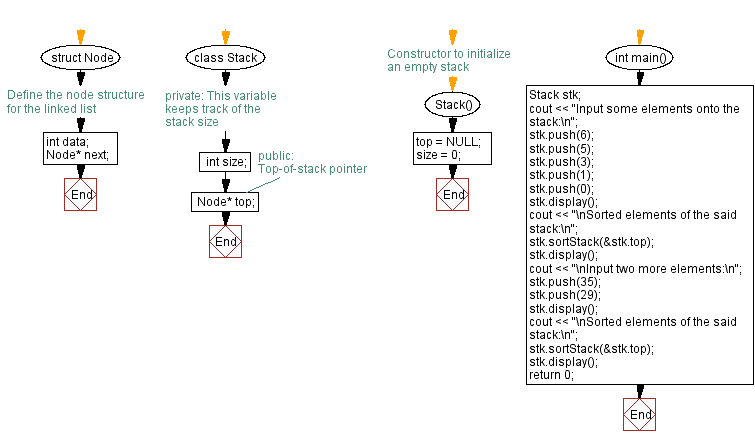
For more Practice: Solve these Related Problems:
- Write a C++ program to sort a linked list-based stack in ascending order and display the sorted result.
- Develop a C++ program that rearranges the elements of a stack (using a linked list) using insertion sort.
- Design a C++ program to implement a linked list-based stack and then sort its elements by modifying the node links.
- Implement a C++ program that sorts a linked list-based stack and prints the stack before and after the sorting operation.
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Reverse the elements of a stack (using a linked list).
Next C++ Exercise: Implement a stack using a dynamic array with push, pop.
What is the difficulty level of this exercise?