C++ Reverse the stack (using a Deque) elements
C++ Stack: Exercise-30 with Solution
Write a C++ program that reverse the stack (using a Deque) elements.
Test Data:
Create a stack object:
Input and store (using Deque) some elements onto the stack:
Stack elements are: 1 3 2 6 5 -1 0
Reverse the stack items in ascending order:
Stack elements are: 0 -1 5 6 2 3 1
Sample Solution:
C++ Code:
#include <iostream>
#include <deque>
#include <algorithm>
using namespace std;
class Stack {
private:
deque<int> elements; // Deque to store elements
public:
// Function to add an element to the stack
void push(int element) {
elements.push_back(element); // Add element to deque
}
// Function to remove an element from the stack
void pop() {
if (elements.empty()) {
cout << "Stack underflow" << endl; // Display underflow message if deque is empty
} else {
elements.pop_back(); // Remove last element from deque
}
}
// Function to get the top element of the stack
int top() {
if (elements.empty()) {
cout << "Stack is empty" << endl; // Display empty message if deque is empty
return 0;
} else {
return elements.back(); // Return last element in deque
}
}
// Function to check if the stack is empty
bool empty() {
return elements.empty(); // Check if deque is empty
}
// Function to reverse elements in the stack
void reverse_elements() {
if (elements.empty()) {
cout << "Stack is empty" << endl; // Display empty message if deque is empty
return;
}
reverse(elements.begin(), elements.end()); // Reverse elements
}
// Function to display the elements in the stack
void display() {
deque<int> d = elements;
if (d.empty()) {
cout << "Stack is empty" << endl; // Display empty message if deque is empty
return;
}
cout << "Stack elements are: ";
for (int i = 0; i < d.size(); i++) {
cout << d[i] << " "; // Display the elements in the deque
}
cout << endl;
}
};
int main() {
Stack stack;
//Initialize a stack
cout << "Create a stack object:\n";
cout << "\nInput and store (using Deque) some elements onto the stack:\n";
stack.push(1);
stack.push(3);
stack.push(2);
stack.push(6);
stack.push(5);
stack.push(-1);
stack.push(0);
stack.display();
cout << "\nReverse the stack items:\n";
stack.reverse_elements();
stack.display();
cout << "\nRemove two elements from the stack:\n";
stack.pop();
stack.pop();
stack.display();
cout << "\nInput three elements onto the stack:\n";
stack.push(4);
stack.push(7);
stack.push(-2);
stack.display();
cout << "\nReverse the said items:\n";
stack.reverse_elements();
stack.display();
return 0;
}
Sample Output:
Create a stack object: Input and store (using Deque) some elements onto the stack: Stack elements are: 1 3 2 6 5 -1 0 Reverse the stack items: Stack elements are: 0 -1 5 6 2 3 1 Remove two elements from the stack: Stack elements are: 0 -1 5 6 2 Input three elements onto the stack: Stack elements are: 0 -1 5 6 2 4 7 -2 Reverse the said items: Stack elements are: -2 7 4 2 6 5 -1 0
Flowchart:
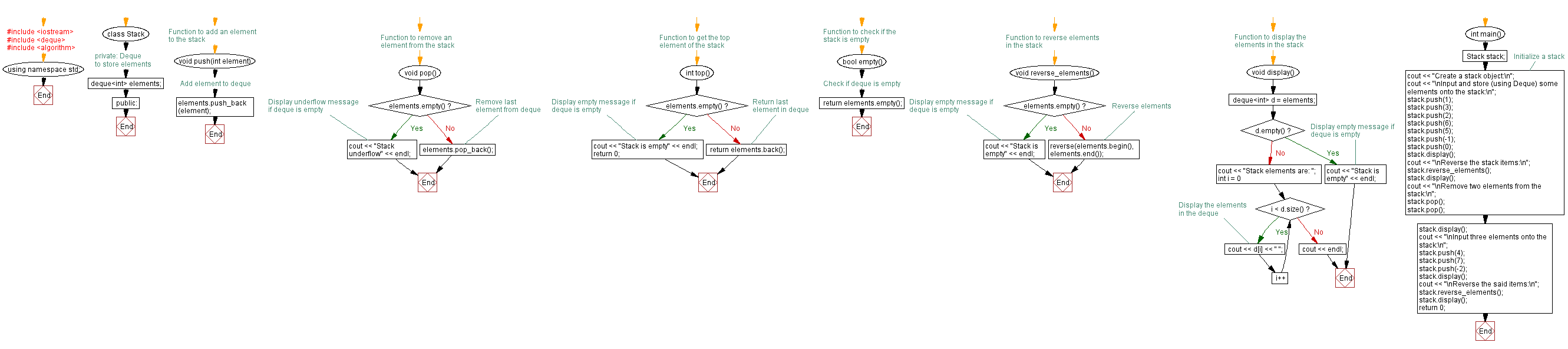
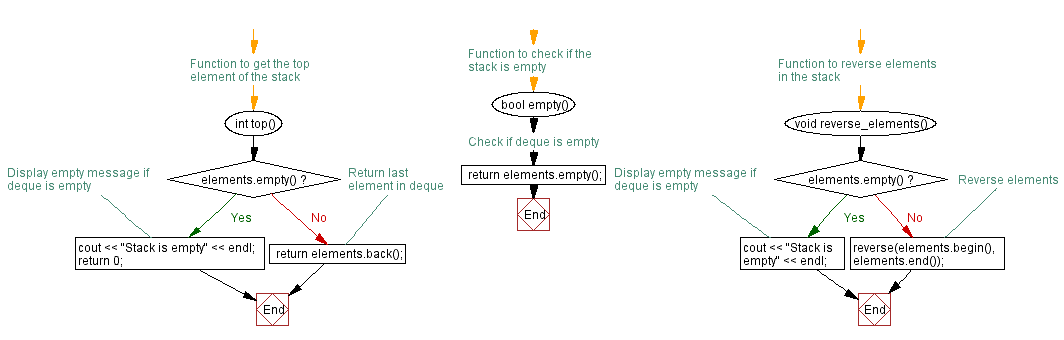
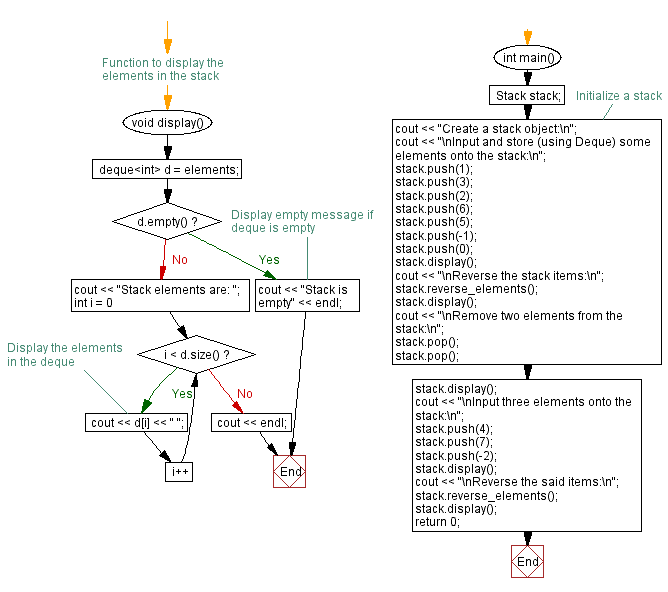
CPP Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Sort the stack (using a Deque) elements.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/stack/cpp-stack-exercise-30.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics