C++ Vector Exercises: Create an n x n matrix by taking an integer (n)
3. Create an n x n Matrix from Input
Write a C++ program to create an n x n matrix by taking an integer (n) as input from the user.
Sample Data:
(2) ->
[ [ 2, 2],
[2, 2]]
(5) ->
[ [ 5,5,5,5,5],
[ 5,5,5,5,5],
[ 5,5,5,5,5],
[ 5,5,5,5,5],
[ 5,5,5,5,5]]
Sample Solution-1:
C++ Code:
#include <algorithm> // Including the Algorithm Library for certain functions like sort
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to create an n x n matrix filled with values equal to 'n'
std::vector<std::vector<int>> test(int n) {
std::vector<int> t(n, n); // Creating a vector 't' of size 'n' filled with 'n'
std::vector<std::vector<int>> arr(n, t); // Creating a 2D vector 'arr' of size 'n x n' filled with vector 't'
return arr; // Returning the 'n x n' matrix
}
// Main function
int main() {
int n;
cout << "Input an integer value: ";
cin >> n; // Taking user input for the size of the matrix
std::vector<std::vector<int>> result = test(n); // Calling the test function to create the 'n x n' matrix
cout << "Create an n x n matrix by said integer:\n";
// Print the elements of the created 'n x n' matrix
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++)
printf("%d ", result[i][j]); // Printing each element of the matrix
printf("\n"); // Moving to the next row after each inner loop completes
}
}
Sample Output:
Input: 2 Input an integer value: Create an n x n matrix by said integer: 2 2 2 2
Flowchart:
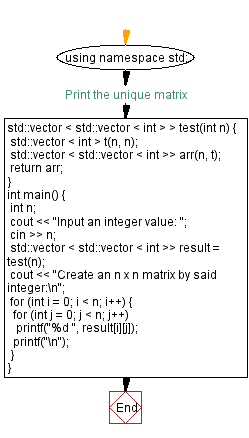
Sample Solution-2:
C++ Code:
#include <algorithm> // Including the Algorithm Library for certain functions like sort
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to create an n x n matrix filled with values equal to 'n'
std::vector<std::vector<int>> test(int n) {
std::vector<int> vect_data(n, n); // Creating a vector 'vect_data' of size 'n' filled with 'n'
std::vector<std::vector<int>> result(n, vect_data); // Creating a 2D vector 'result' of size 'n x n' filled with vector 'vect_data'
return result; // Returning the 'n x n' matrix
}
// Main function
int main() {
int n;
cout << "Input an integer value: ";
cin >> n; // Taking user input for the size of the matrix
std::vector<std::vector<int>> result = test(n); // Calling the test function to create the 'n x n' matrix
cout << "Create an n x n matrix by said integer:\n";
// Print the elements of the created 'n x n' matrix
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++)
printf("%d ", result[i][j]); // Printing each element of the matrix
printf("\n"); // Moving to the next row after each inner loop completes
}
}
Sample Output:
Input: 5 Input an integer value: Create an n x n matrix by said integer: 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5 5
Flowchart:
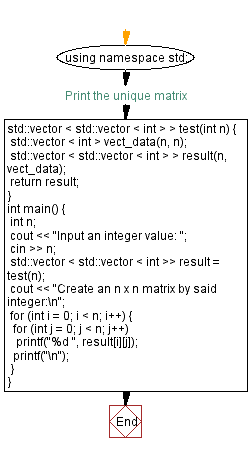
For more Practice: Solve these Related Problems:
- Write a C++ program that reads an integer n and creates an n x n matrix filled with the same input value using nested loops.
- Write a C++ program to generate an n x n matrix where each element is the product of its row and column indices.
- Write a C++ program that constructs an n x n identity matrix based on a user-provided integer n.
- Write a C++ program to create an n x n matrix where each element is calculated as a function of n, its row, and column positions, and print the matrix.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Vector elements smaller than its adjacent neighbours.
Next C++ Exercise: Capitalize the first character of each vector element.
What is the difficulty level of this exercise?