C++ Vector Exercises: Capitalize the first character of each vector element
C++ Vector: Exercise-4 with Solution
Write a C++ program to capitalize the first character of each element of a given string vector. Return the new vector.
Sample Data:
({"red", "green", "black", "white", "Pink"}) ->
("Red", "Green", "Black", "White", "Pink"})
Sample Solution-1:
C++ Code:
#include <algorithm> // Including the Algorithm Library for certain functions like sort
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to modify the vector of strings by capitalizing the first character of each word and converting the rest to lowercase
std::vector<std::string> test(std::vector<std::string> colors) {
for(int i = 0; i < colors.size(); i++) { // Looping through each string in the vector
colors[0][0] = toupper(colors[0][0]); // Capitalizing the first character of the first string in the vector
// Capitalizing the first character of each string in the vector and converting the rest to lowercase
colors[i][0] = toupper(colors[i][0]); // Capitalizing the first character of the current string
for(int l = 1; l < colors[i].size(); l++) { // Looping through the characters of the current string (except the first character)
colors[i][l] = tolower(colors[i][l]); // Converting characters to lowercase
}
}
return colors; // Returning the modified vector of strings
}
// Main function
int main() {
vector<string> colors = {"red", "green", "black", "white", "Pink"}; // Initializing a vector of strings
cout << "Original Vector elements:\n";
for (string c : colors)
cout << c << " "; // Printing the original elements of the vector
vector<string> result = test(colors); // Calling the test function to modify the strings in the vector
cout << "\n\nCapitalize the first character of each vector element:\n";
for (string c : result)
cout << c << " "; // Printing the modified elements of the vector
}
Sample Output:
Original Vector elements: red green black white Pink Capitalize the first character of each vector element: Red Green Black White Pink
Flowchart:
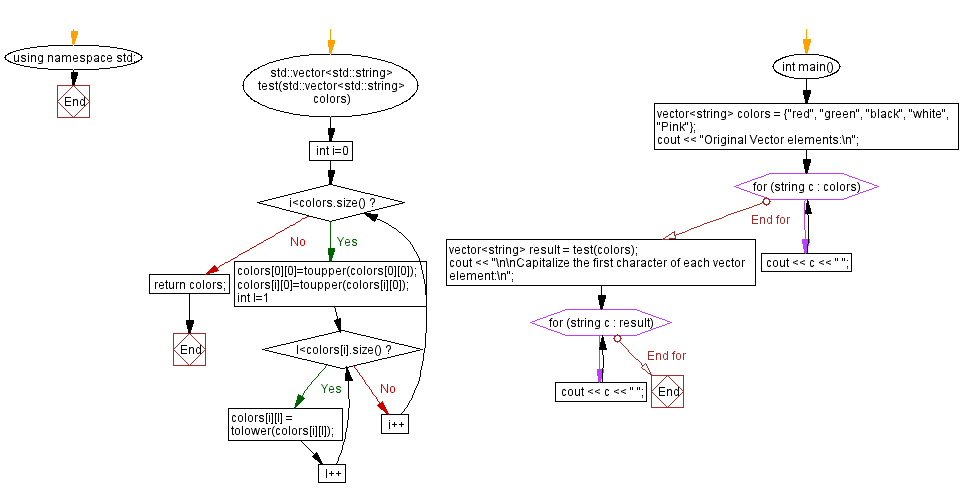
Sample Solution-2:
C++ Code:
#include <algorithm> // Including the Algorithm Library for certain functions like transform
#include <iostream> // Including the Input/Output Stream Library
#include <vector> // Including the Vector Library for using vectors
using namespace std; // Using the Standard Namespace
// Function to modify the vector of strings by capitalizing the first character of each word and converting the rest to lowercase
std::vector<std::string> test(std::vector<std::string> colors) {
for (std::string &cl : colors) { // Looping through each string in the vector by reference
std::transform(cl.begin(), cl.end(), cl.begin(), ::tolower); // Converting all characters in the string to lowercase
cl[0] = std::toupper(cl[0]); // Capitalizing the first character of the string
}
return colors; // Returning the modified vector of strings
}
// Main function
int main() {
vector<string> colors = {"red", "green", "black", "white", "Pink"}; // Initializing a vector of strings
cout << "Original Vector elements:\n";
for (string c : colors)
cout << c << " "; // Printing the original elements of the vector
vector<string> result = test(colors); // Calling the test function to modify the strings in the vector
cout << "\n\nCapitalize the first character of each vector element:\n";
for (string c : result)
cout << c << " "; // Printing the modified elements of the vector
}
Sample Output:
Original Vector elements: red green black white Pink Capitalize the first character of each vector element: Red Green Black White Pink
Flowchart:
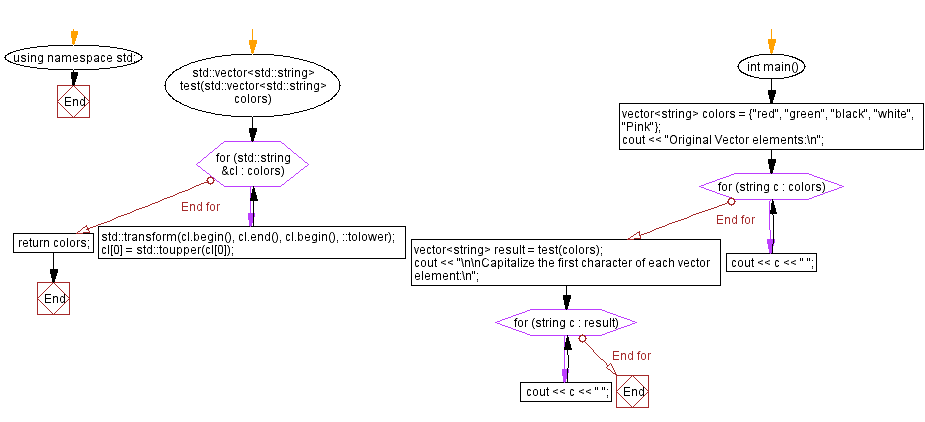
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous C++ Exercise: Create an n x n matrix by taking an integer (n).
Next C++ Exercise: First string contains all letters from second string.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/cpp-exercises/vector/cpp-vector-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics