C#: Remove all duplicate elements from a given array
Write a C# Sharp program that removes all duplicate elements from a given array and returns an updated array.
Sample Solution:-
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
// Create an array of objects containing various data types
object[] mixedArray = new object[8];
mixedArray[0] = 25;
mixedArray[1] = "Anna";
mixedArray[2] = false;
mixedArray[3] = 25;
mixedArray[4] = System.DateTime.Now;
mixedArray[5] = 112.22;
mixedArray[6] = "Anna";
mixedArray[7] = false;
// Display the original elements in the mixed array
Console.WriteLine("Original array elements:");
for (int i = 0; i < mixedArray.Length; i++)
{
Console.WriteLine(mixedArray[i]);
}
// Call the 'test' method to remove duplicates from the mixed array
object[] result = test(mixedArray);
// Display the elements after removing duplicates from the array
Console.WriteLine("\nAfter removing duplicate elements from the said array:");
for (int i = 0; i < result.Length; i++)
{
Console.WriteLine(result[i]);
}
}
// Method to remove duplicate elements from an array of objects
public static object[] test(object[] mixedArray)
{
// Use LINQ's 'Distinct' method to filter out duplicate elements and convert the result back to an array
return mixedArray.Distinct().ToArray();
}
}
}
Sample Output:
Original array elements: 25 Anna False 25 4/15/2021 12:10:51 PM 112.22 Anna False After removing duplicate elements from the said array: 25 Anna False 4/15/2021 12:10:51 PM 112.22
Flowchart:
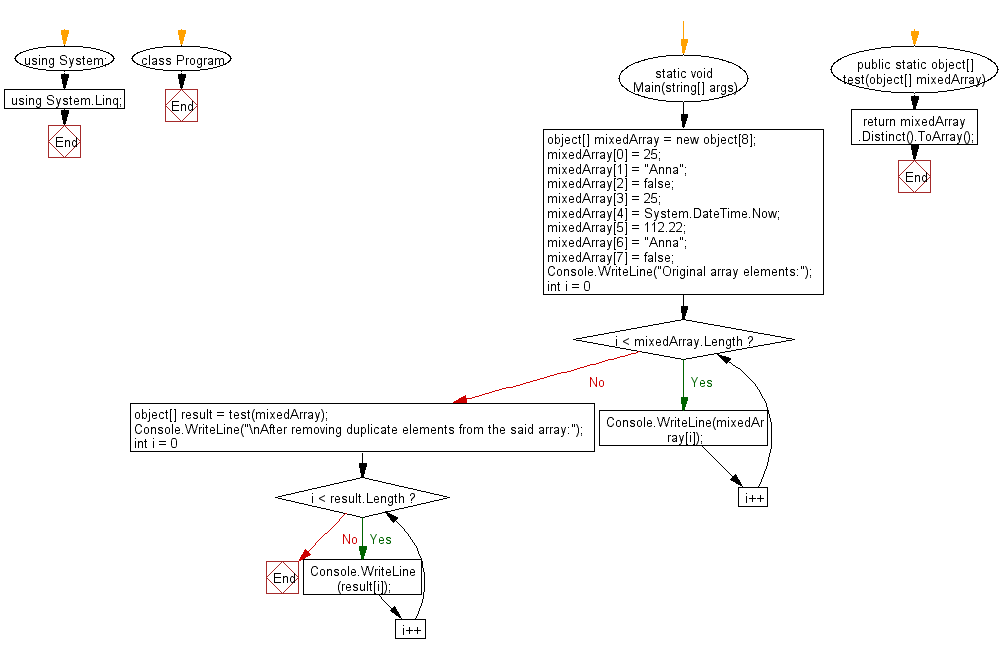
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to get only the odd values from a given array of integers.
Next: Write a C# Sharp program to find the missing number in a given array of numbers between 10 and 20.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.