C#: Check whether a given positive number is a multiple of 3 or a multiple of 7
Multiple of 3 or 7
Write a C# Sharp program to check if a given positive number is a multiple of 3 or 7.
Visual Presentation:
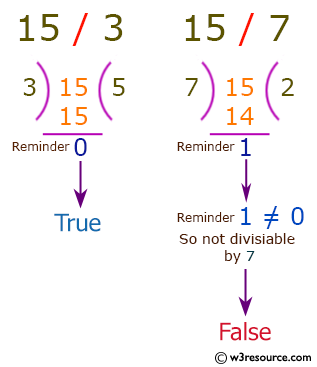
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and displaying the returned boolean values
Console.WriteLine(test(3)); // Output: True
Console.WriteLine(test(14)); // Output: True
Console.WriteLine(test(12)); // Output: True
Console.WriteLine(test(37)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to check if an integer is divisible by 3 or 7
public static bool test(int n)
{
// Returns true if the input integer is divisible by 3 or 7
return n % 3 == 0 || n % 7 == 0;
}
}
}
Sample Output:
True True True False
Flowchart:
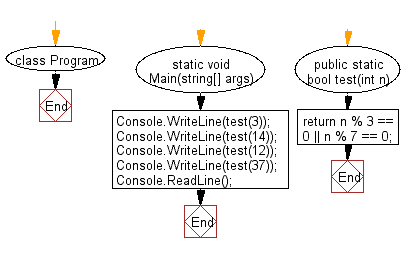
For more Practice: Solve these Related Problems:
- Write a C# program to return true if a number is divisible by both 3 and 7, but not by 2.
- Write a C# program to check if a number is a multiple of 3, 5, or 7 and print a different word for each.
- Write a C# program to test whether a number is a power of 3 or a multiple of 7.
- Write a C# program to return true only if a number is divisible by 3 or 7 but not both.
PREV : Last Character at Front and Back.
NEXT : First Three Characters Front and Back.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.