C#: Create a new string taking the first 3 characters of a given string and return the string with the 3 characters added at both the front and back
First Three Characters Front and Back
Write a C# Sharp program to create a string taking the first 3 characters of a given string. Return the string with the 3 characters added at both the front and back. If the given string length is less than 3, use whatever characters are there.
Visual Presentation:
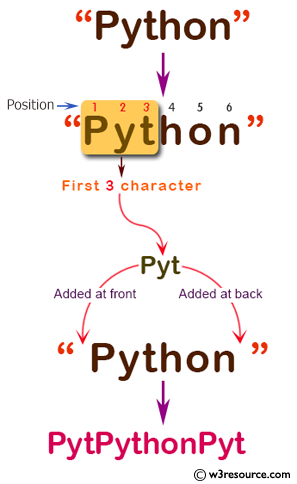
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings and displaying the returned values
Console.WriteLine(test("Python")); // Output: PytPythonPyt
Console.WriteLine(test("JS")); // Output: JSJSJS
Console.WriteLine(test("Code")); // Output: CodCodeCod
Console.ReadLine(); // Keeping the console window open
}
// Method to process a string based on its length
public static string test(String str)
{
// If the length of the string is less than 3, concatenate the string with itself twice
if (str.Length < 3)
{
return str + str + str;
}
else
{
// If the length of the string is 3 or more, extract the first three characters
string front = str.Substring(0, 3);
// Concatenate the first three characters, the original string, and the first three characters again
return front + str + front;
}
}
}
}
Sample Output:
PytPythonPyt JSJSJS CodCodeCod
Flowchart:
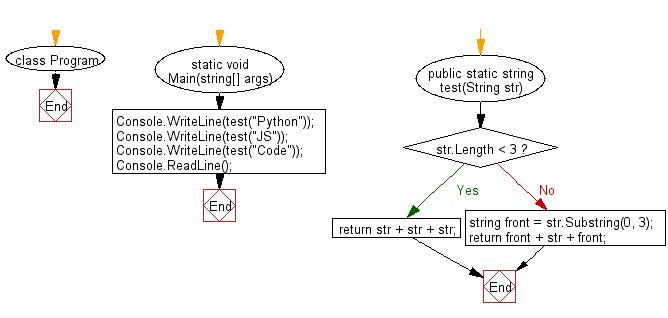
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if a given positive number is a multiple of 3 or a multiple of 7.
Next: Write a C# Sharp program to check if a given string starts with 'C#' or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.