C#: Check whether a given string starts with 'C#' or not
Starts with 'C#'
Write a C# Sharp program to check whether a given string starts with 'C#' or not.
Visual Presentation:
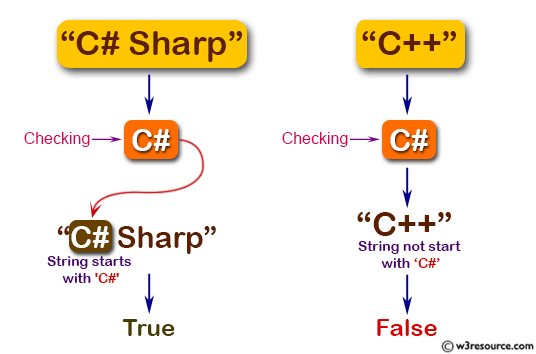
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different strings and displaying the returned values
Console.WriteLine(test("C# Sharp")); // Output: True
Console.WriteLine(test("C#")); // Output: True
Console.WriteLine(test("C++")); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to test conditions based on the given string
public static bool test(string str)
{
// Check if the length of the string is less than 3 and it equals "C#"
// or if the string starts with "C#" and the third character is a space ' '
return (str.Length < 3 && str.Equals("C#")) || (str.StartsWith("C#") && str[2] == ' ');
}
}
}
Sample Output:
True True False
Flowchart:
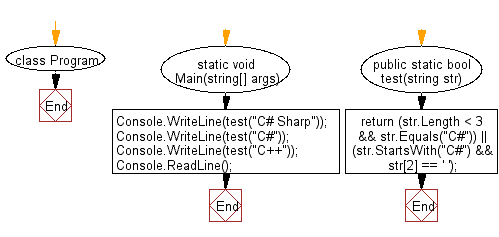
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new string taking the first 3 characters of a given string and return the string with the 3 characters added at both the front and back.If the given string length is less than 3, use whatever characters are there.
Next: Write a C# Sharp program to check if one given temperatures is less than 0 and the other is greater than 100.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.