C#: Check whether one of the given temperatures is less than 0 and the other is greater than 100
C# Sharp Basic Algorithm: Exercise-13 with Solution
Temperature Comparison
Write a C# Sharp program that checks whether one temperature is less than 0 and another is greater than 100.
Visual Presentation:
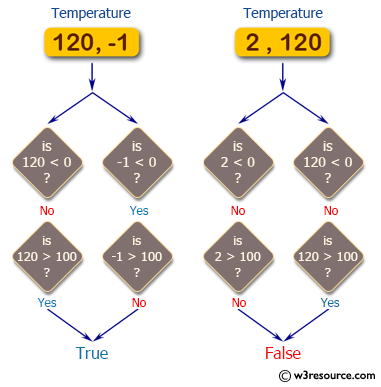
Sample Solution:
C# Sharp Code:
using System;
// Namespace declaration
namespace exercises
{
// Class declaration
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Calling the 'test' method with different integers and displaying the returned values
Console.WriteLine(test(120, -1)); // Output: True
Console.WriteLine(test(-1, 120)); // Output: True
Console.WriteLine(test(2, 120)); // Output: False
Console.ReadLine(); // Keeping the console window open
}
// Method to test conditions based on two integer values
public static bool test(int temp1, int temp2)
{
// Check if temp1 is less than 0 AND temp2 is greater than 100
// OR check if temp2 is less than 0 AND temp1 is greater than 100
// Return true if either of these conditions is met; otherwise, return false
return temp1 < 0 && temp2 > 100 || temp2 < 0 && temp1 > 100;
}
}
}
Sample Output:
True True False
Flowchart:
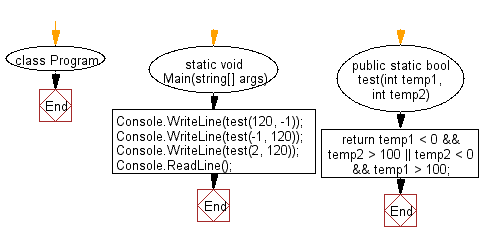
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if a given string starts with "C#" or not.
Next: Write a C# Sharp program to check two given integers whether either of them is in the range 100..200 inclusive.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics