C#: Check a given array of integers, length 3 and create a new array
Set 7 to 1 if Followed by 5
Write a C# Sharp program to check a given array of integers length 3 and create a array. If there is a 5 in the given array immediately followed by a 7 then set 7 to 1.
Visual Presentation:
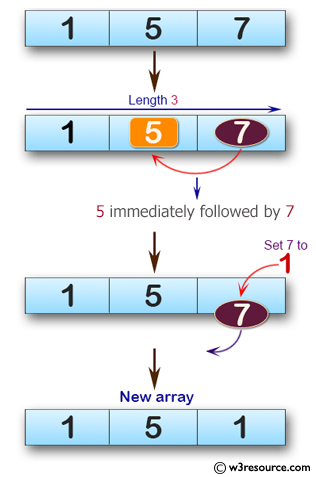
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an integer array as an argument and assigning the result to the 'item' array
int[] item = test(new[] { 1, 5, 7 });
// Outputting text to indicate the start of displaying the new array
Console.Write("New array: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to replace the element following 5 with 1, if 5 is followed by 7 in the input array
static int[] test(int[] numbers)
{
for (var i = 0; i < numbers.Length - 1; i++)
{
if (numbers[i].Equals(5) && numbers[i + 1].Equals(7))
numbers[i + 1] = 1; // If 5 is followed by 7, replace the following element with 1
}
return numbers; // Returning the modified array
}
}
}
Sample Output:
New array: 1 5 1
Flowchart:
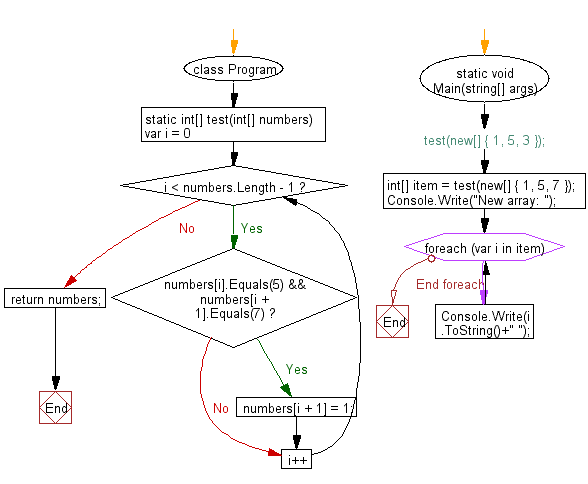
Go to:
PREV : Check if 10 or 20 Appears Twice in Array.
NEXT : Largest Sum Between Two Arrays.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.