C#: Check a given array of integers and return true if the array contains 10 or 20 twice
Check if 10 or 20 Appears Twice in Array
Write a C# Sharp program to check a given array of integers and return true if it contains 10 or 20 twice. The array length will be 0, 1, or 2.
Visual Presentation:
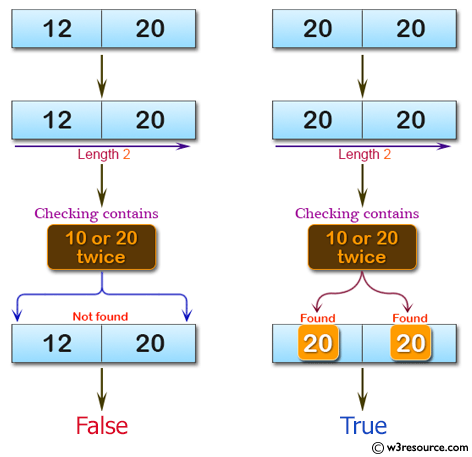
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 12, 20 })); // Calling test method with array [12, 20] and displaying the result
Console.WriteLine(test(new[] { 20, 20 })); // Calling test method with array [20, 20] and displaying the result
Console.WriteLine(test(new[] { 10, 10 })); // Calling test method with array [10, 10] and displaying the result
Console.WriteLine(test(new[] { 10 })); // Calling test method with array [10] and displaying the result
}
// Method to check if the input array has a length of 2 and contains either two 10s or two 20s
public static bool test(int[] nums)
{
// Checking if the array length is equal to 2
// and if the elements are either both 10 or both 20
return nums.Length == 2
&& ((nums[0] == 10 && nums[1] == 10)
|| (nums[0] == 20 && nums[1] == 20));
}
}
}
Sample Output:
False True True False
Flowchart:
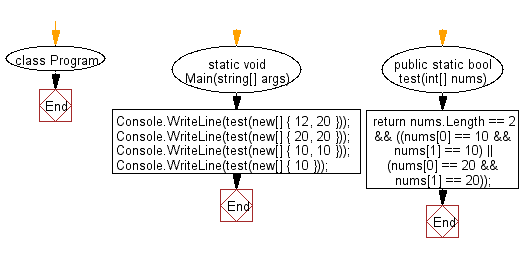
Go to:
PREV : Double Length Array with First Element Copied.
NEXT : Set 7 to 1 if Followed by 5.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.