C#: Create a new array of integers and length 1 or more
Double Length Array with First Element Copied
Write a C# Sharp program to create an array of integers with a length of 1 or more. The array length will be double the given array length. All the elements are 0 except the first element which is equal to the given array.
Visual Presentation:
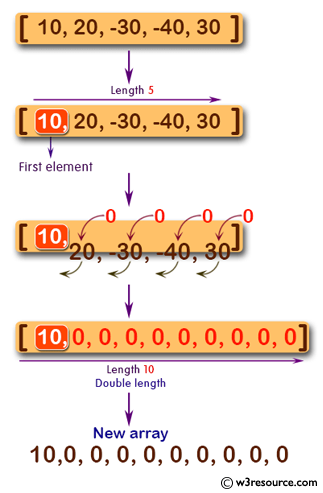
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an integer array as an argument and assigning the result to the 'item' array
int[] item = test(new[] { 10, 20, -30, -40, 30 });
// Outputting text to indicate the start of displaying the new array
Console.Write("New array: ");
// Looping through each element of the 'item' array and displaying them
foreach (var i in item)
{
Console.Write(i.ToString() + " "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to create a new array with double the length of the input array and copies the first element into the new array
public static int[] test(int[] nums)
{
int elements = nums.Length * 2; // Calculating the number of elements needed for the new array
int[] doubleNums = new int[elements]; // Creating a new array with double the length of the input array
doubleNums[0] = nums[0]; // Copying the first element of 'nums' to the first element of 'doubleNums'
return doubleNums; // Returning the new array 'doubleNums'
}
}
}
Sample Output:
New array: 10 0 0 0 0 0 0 0 0 0
Flowchart:
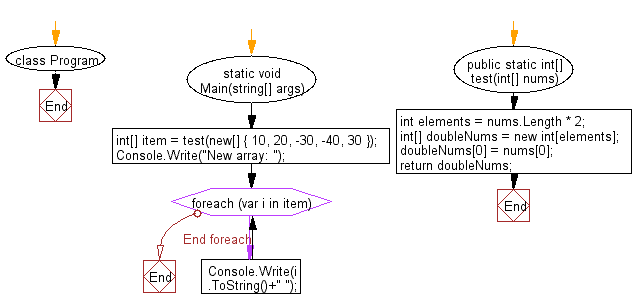
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if a given array of integers and length 2, does not contain 15 or 20
Next: Write a C# Sharp program to check a given array of integers and return true if the array contains 10 or 20 twice. The length of the array will be 0, 1, or 2.s
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.