C#: Create a new array swapping the first and last elements of a given array of integers and length will be least 1
Swap First and Last Array Elements
Write a C# Sharp program to create an array by swapping the first and last elements of a given array of integers with a length of at least 1.
Visual Presentation:
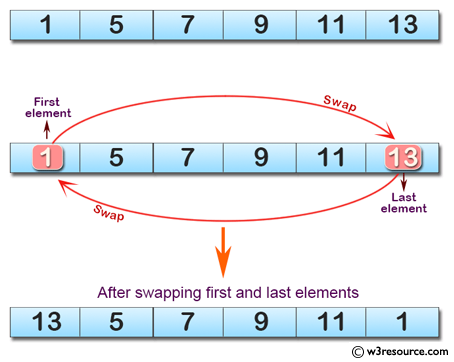
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an integer array as an argument and assigning the result to the 'item' array
int[] item = test(new[] { 1, 5, 7, 9, 11, 13 });
// Outputting text to indicate the start of displaying the result after swapping
Console.Write("After swapping first and last elements: ");
// Looping through each element of the 'item' array and displaying them
foreach(var i in item)
{
Console.Write(i.ToString()+" "); // Displaying each element of the 'item' array separated by a space
}
}
// Method to swap the first and last elements of the input array
static int[] test(int[] numbers)
{
int first = numbers[0]; // Storing the value of the first element in a temporary variable
numbers[0] = numbers[numbers.Length - 1]; // Assigning the value of the last element to the first element
numbers[numbers.Length - 1] = first; // Assigning the value of the temporary variable to the last element
return numbers; // Returning the modified array
}
}
}
Sample Output:
After swapping first and last elements: 13 5 7 9 11 1
Flowchart:
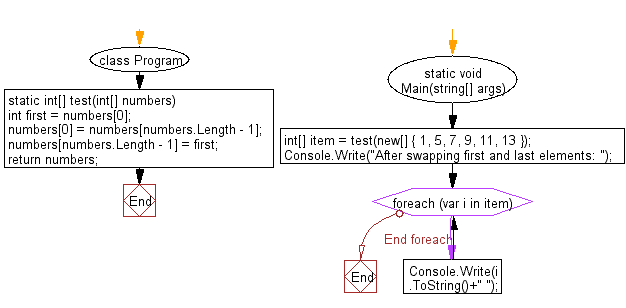
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array from two give array of integers, each length 3.
Next: Write a C# Sharp program to create a new array length 4 from a given array (length atleast 4) containing the elements from the middle of the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.