C#: Check whether a given array of integers contains 5 next to a 5 somewhere
C# Sharp Basic Algorithm: Exercise-113 with Solution
Check for 5 Next to 5
Write a C# Sharp program to check whether a given array of integers contains 5 next to a 5 somewhere.
Visual Presentation:
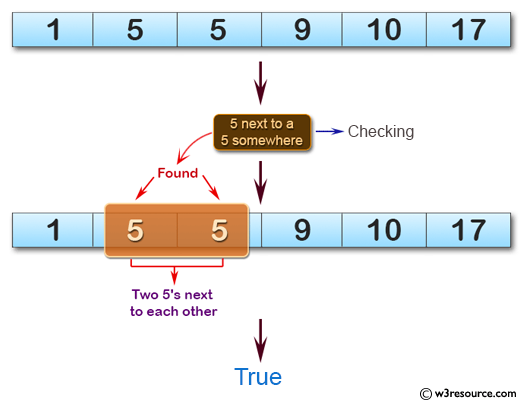
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 1, 5, 6, 9, 10, 17 })); // Testing the method with an array
Console.WriteLine(test(new[] { 1, 5, 5, 9, 10, 17 })); // Testing the method with another array
Console.WriteLine(test(new[] { 1, 5, 5, 9, 10, 17, 5, 5 })); // Testing the method with a third array
}
// Method to check if there are adjacent occurrences of the number 5 in the array
static bool test(int[] nums)
{
// Looping through the array except for the last element
for (int i = 0; i < nums.Length - 1; i++)
{
// Checking if the current element is 5 and if the next element is also 5
if (nums[i] == 5 && nums[i] == nums[i + 1])
{
return true; // If two consecutive 5s are found, returning true
}
}
return false; // If no consecutive 5s are found, returning false
}
}
}
Sample Output:
False True True
Flowchart:
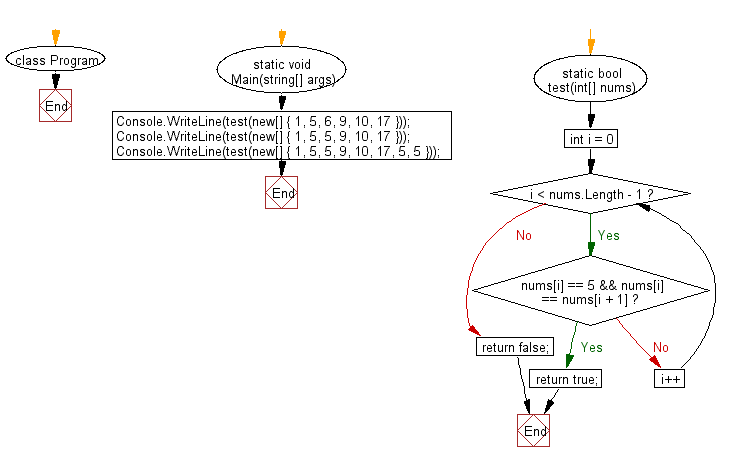
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compute the sum of the numbers in a given array except those numbers starting with 5 followed by at least one 6. Return 0 if the given array has no integer.
Next: Write a C# Sharp program to check whether a given array of integers contains 5's and 7's.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-113.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics