C#: Check whether a given array of integers contains 5's or 7's
Check for Both 5's and 7's in Array
Write a C# Sharp program to check whether a given array of integers contains 5's or 7's.
Visual Presentation:
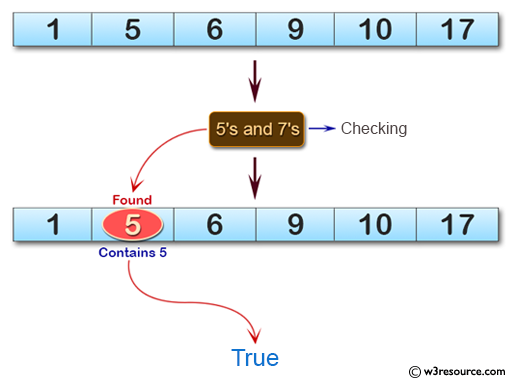
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 1, 5, 6, 9, 10, 17 })); // Testing the method with an array
Console.WriteLine(test(new[] { 1, 4, 7, 9, 10, 17 })); // Testing the method with another array
Console.WriteLine(test(new[] { 1, 1, 2, 9, 10, 17 })); // Testing the method with a third array
}
// Method to check if the array contains either the number 5 or the number 7
static bool test(int[] nums)
{
// Looping through the array
for (int i = 0; i < nums.Length; i++)
{
// Checking if the current element is either 5 or 7
if (nums[i] == 5 || nums[i] == 7)
{
return true; // If 5 or 7 is found, returning true
}
}
return false; // If neither 5 nor 7 is found, returning false
}
}
}
Sample Output:
True True False
Flowchart:
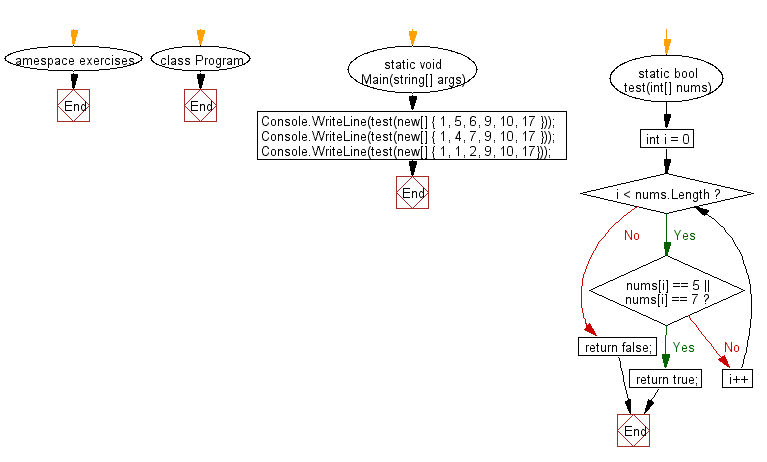
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check if a given array of integers contains 5 next to a 5 somewhere.
Next: Write a C# Sharp program to check if the sum of all 5' in the array exactly 15 in a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.