C#: Check a given array of integers and return true if the value 5 appears 5 times and there are no 5 next to each other
Check for 5 Exactly Five Times Without Adjacent
Write a C# Sharp program to check a given array of integers and return true if 5 appears 5 times. There are no 5 next to each other.
Visual Presentation:
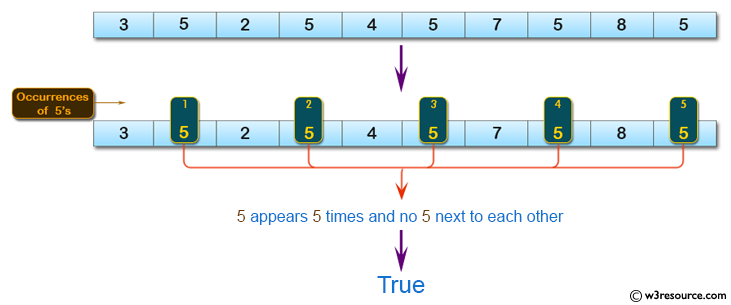
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace CheckArray // Defining a namespace called 'CheckArray'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the results of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 3, 5, 1, 5, 3, 5, 7, 5, 1, 5 })); // Testing the method with various arrays
Console.WriteLine(test(new[] { 3, 5, 5, 5, 5, 5, 5 })); // Testing the method with various arrays
Console.WriteLine(test(new[] { 3, 5, 2, 5, 4, 5, 7, 5, 8, 5 })); // Testing the method with various arrays
Console.WriteLine(test(new[] { 2, 4, 5, 5, 5, 5 })); // Testing the method with various arrays
Console.WriteLine(test(new[] { 3, 5, 5, 5, 5, 5, 5, 5, 5, 5 })); // Testing the method with various arrays
}
// Method to check if the array contains exactly five occurrences of '5', with no adjacent '5's
static bool test(int[] arr)
{
int ctr = 0; // Counter to track the occurrences of '5'
for (int i = 0; i < arr.Length; i++) // Looping through the elements of the array
{
if (arr[i] == 5) // Checking if the element is '5'
{
ctr++; // Incrementing the counter for each occurrence of '5'
// Checking if the next element is also '5' when '5' is found
if (i < arr.Length - 1 && arr[i + 1] == 5)
{
return false; // Returning false if consecutive '5's are found
}
}
}
// Returning true if exactly five occurrences of '5' are found with no consecutive '5's
return ctr == 5;
}
}
}
Sample Output:
True False True False False
Flowchart:
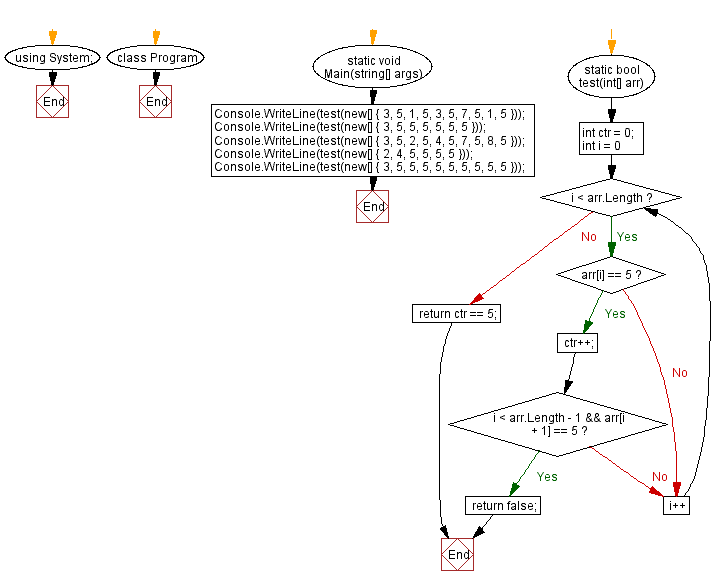
Go to:
PREV : Two Consecutive Even or Odd Values.
NEXT : Check If Every 5 is Next to Another 5.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.