C#: Check a given array of integers and return true if every 5 that appears in the given array is next to another 5
Check If Every 5 is Next to Another 5
Write a C# Sharp program to check a given array of integers. Return true if every 5 in the given array is next to another 5.
Visual Presentation:
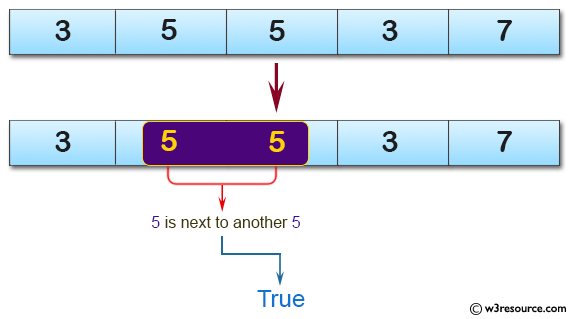
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the results of the 'test' method with different integer arrays as arguments
Console.WriteLine(test(new[] { 3, 5, 5, 3, 7 })); // Testing the method with various arrays
Console.WriteLine(test(new[] { 3, 5, 5, 4, 1, 5, 7 })); // Testing the method with various arrays
Console.WriteLine(test(new[] { 3, 5, 5, 5, 5, 5 })); // Testing the method with various arrays
Console.WriteLine(test(new[] { 2, 4, 5, 5, 6, 7, 5 })); // Testing the method with various arrays
}
// Method to check if '5' exists in the array but not adjacent to another '5'
static bool test(int[] numbers)
{
int arr_len = numbers.Length; // Storing the length of the array
bool flag = true; // Initializing a flag to true
for (int i = 0; i < arr_len; i++) // Looping through the elements of the array
{
if (numbers[i] == 5) // Checking if the current element is '5'
{
if ((i > 0 && numbers[i - 1] == 5) || (i < arr_len - 1 && numbers[i + 1] == 5)) // Checking if '5' is adjacent to another '5'
flag = true; // Setting the flag to true if '5' is adjacent to another '5'
else if (i == arr_len - 1) // Checking if '5' is the last element in the array
flag = false; // Setting the flag to false if '5' is the last element and not adjacent to another '5'
else
return false; // Returning false if '5' is not adjacent to another '5'
}
}
return flag; // Returning the flag value
}
}
}
Sample Output:
True False True False
Flowchart:
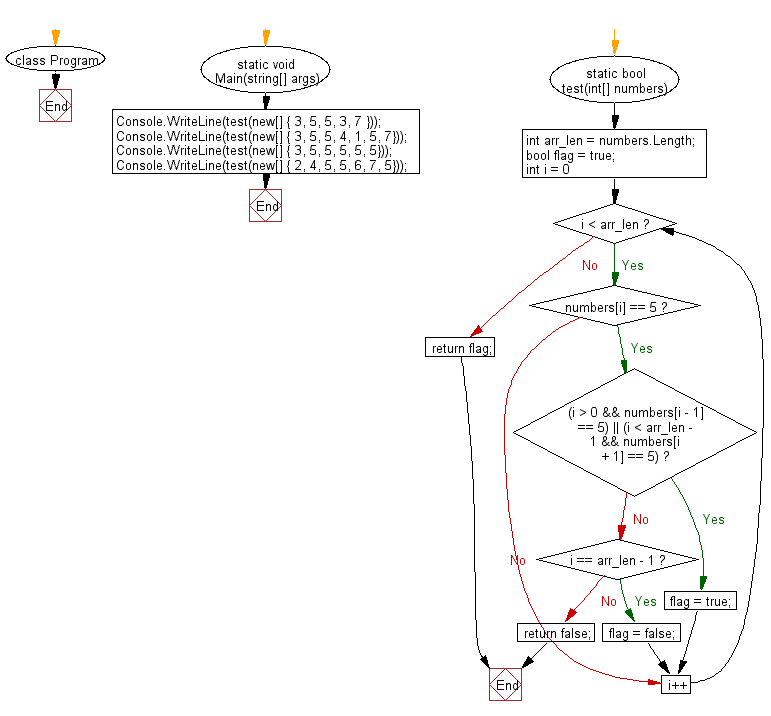
Go to:
PREV : Check for 5 Exactly Five Times Without Adjacent.
NEXT : Check Equal Elements at Start and End.
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.