C#: Check a given array of integers and return true if the specified number of same elements appears at the start and end of the given array
Check Equal Elements at Start and End
Write a C# Sharp program to check a given array of integers. Return true if the specified number of same elements appears at the start and end of the given array.
Visual Presentation:
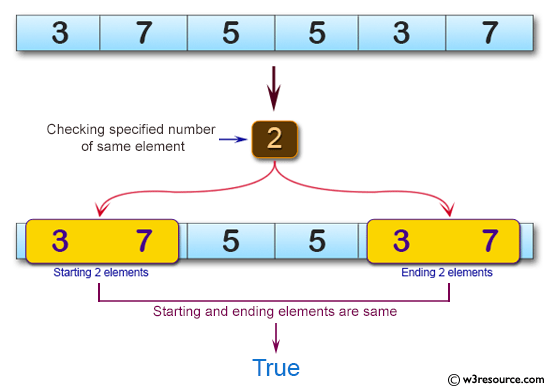
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the results of the 'test' method with different integer arrays and lengths as arguments
Console.WriteLine(test(new[] { 3, 7, 5, 5, 3, 7 }, 2)); // Testing the method with an array and a length
Console.WriteLine(test(new[] { 3, 7, 5, 5, 3, 7 }, 3)); // Testing the method with an array and a length
Console.WriteLine(test(new[] { 3, 7, 5, 5, 3, 7, 5 }, 3)); // Testing the method with an array and a length
}
// Method to check if the first 'len' elements in the array are the same as the last 'len' elements
static bool test(int[] numbers, int len)
{
int arra_size = numbers.Length; // Storing the length of the array
for (int i = 0; i < len; i++) // Looping through the first 'len' elements
{
// Checking if the current element from the beginning and end of the array are different
if (numbers[i] != numbers[arra_size - len + i])
{
return false; // Returning false if any pair of elements are not equal
}
}
return true; // Returning true if all 'len' pairs of elements are equal
}
}
}
Sample Output:
True False True
Flowchart:
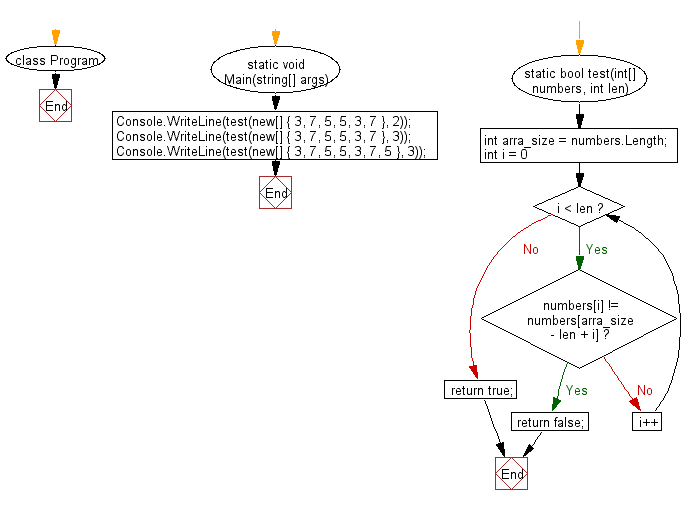
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a given array of integers and return true if every 5 that appears in the given array is next to another 5.
Next: Write a C# Sharp program to check a given array of integers and return true if the array contains three increasing adjacent numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.