C#: Create a new array taking the elements after the element value 5 from a given array of integers
C# Sharp Basic Algorithm: Exercise-129 with Solution
Elements After 5 in Array
Write a C# Sharp program to create an array by taking the elements after element value 5 from a given array of integers.
Visual Presentation:
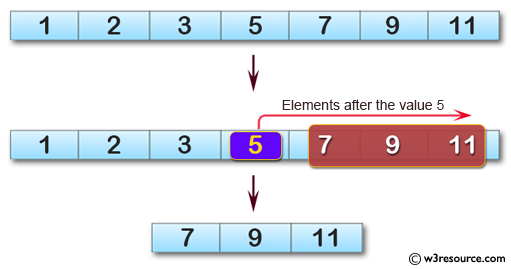
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a given integer array and storing the returned array in 'item'
int[] item = test(new[] { 1, 2, 3, 5, 7, 9, 11 });
// Outputting the elements of the new array formed after the element '5' if found
Console.Write("New array: ");
foreach (var i in item) // Looping through each element in the 'item' array
{
Console.Write(i.ToString() + " "); // Outputting each element followed by a space
}
}
// Method to extract elements of an array after the occurrence of the value '5'
static int[] test(int[] numbers)
{
int len = numbers.Length; // Storing the length of the array
int size = 0; // Variable to store the size of elements after '5'
int[] post_ele_5; // Array to hold elements after '5'
int i = len - 1; // Starting from the last index of the array
// Finding the index of the first occurrence of '5' from the end of the array
while (i >= 0 && numbers[i] != 5)
{
i--;
}
i++; // Incrementing to move to the element after '5'
size = len - i; // Calculating the number of elements after '5'
post_ele_5 = new int[size]; // Creating an array with size determined after '5'
// Storing elements after '5' into the post_ele_5 array
for (int j = 0; j < size; j++)
{
post_ele_5[j] = numbers[i + j];
}
return post_ele_5; // Returning the array containing elements after '5'
}
}
}
Sample Output:
New array: 7 9 11
Flowchart:
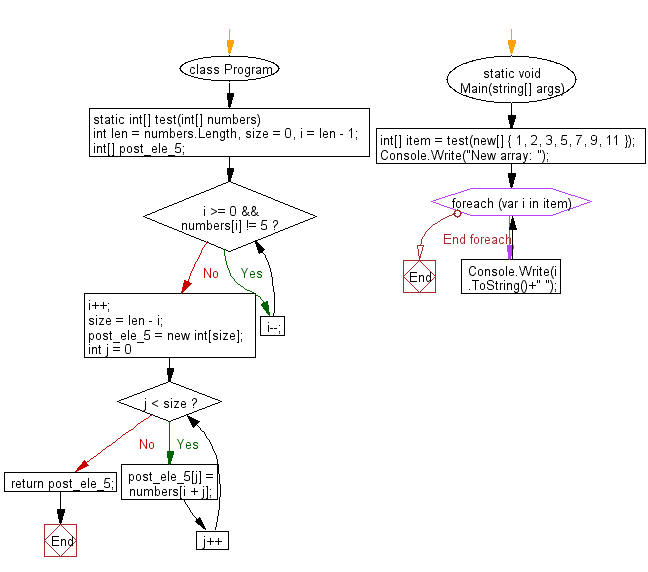
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array taking the elements before the element value 5 from a given array of integers.
Next: Write a C# Sharp program to create a new array from a given array of integers shifting all zeros to left direction.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-129.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics