C#: Create a new array from a given array of integers shifting all zeros to left direction by swapping with number
Shift Zeros to Left in Array
Write a C# Sharp program to create an array from a given array of integers by shifting all zeros to the left direction.
Visual Presentation:
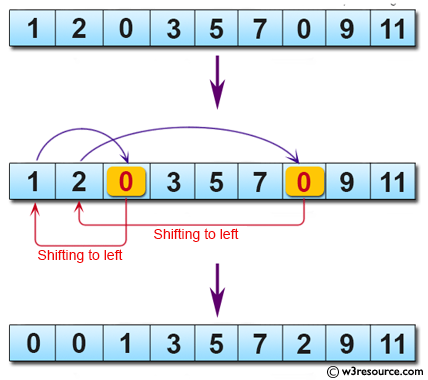
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a given integer array
// and storing the returned modified array in 'item'
int[] item = test(new[] { 1, 2, 0, 3, 5, 7, 0, 9, 11 });
// Outputting the elements of the modified array
Console.Write("New array: ");
foreach (var i in item) // Looping through each element in the 'item' array
{
Console.Write(i.ToString() + " "); // Outputting each element followed by a space
}
}
// Method to move all occurrences of '0' to the end of the array
static int[] test(int[] numbers)
{
int pos = 0; // Variable to track the position of non-zero elements
// Looping through the array to move all '0's to the end
for (int i = 0; i < numbers.Length; i++)
{
if (numbers[i] == 0)
{
// Swapping '0' with the non-zero element at position 'pos'
numbers[i] = numbers[pos]; // Placing non-zero element in place of '0'
numbers[pos++] = 0; // Placing '0' at the next position after the non-zero element
}
}
return numbers; // Returning the modified array
}
}
}
Sample Output:
New array: 0 0 1 3 5 7 2 9 11
Flowchart:
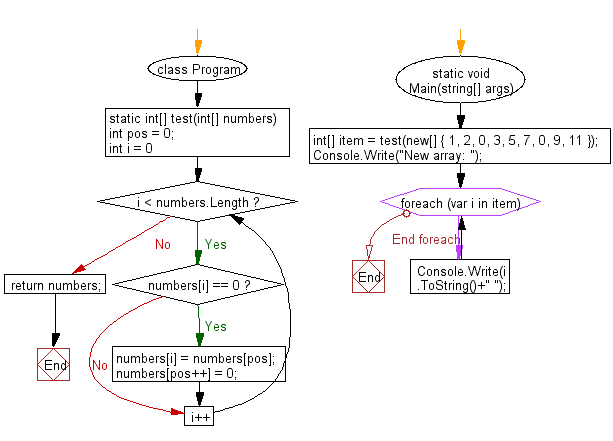
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array taking the elements after the element value 5 from a given array of integers.
Next: Write a C# Sharp program to create a new array after replacing all the values 5 with 0 shifting all zeros to right direction.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.