C#: Create a new array after replacing all the values 5 with 0 and shifting all zeros to right direction
Replace 5 with 0 and Shift Zeros Right
Write a C# Sharp program to create an array after replacing all values 5 with 0 and shifting all zeros to the right.
Visual Presentation:
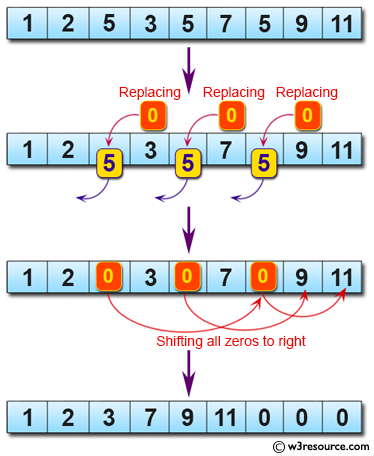
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a given integer array
// and storing the returned modified array in 'item'
int[] item = test(new[] { 1, 2, 5, 3, 5, 7, 5, 9, 11 });
// Outputting the elements of the modified array
Console.Write("New array: ");
foreach (var i in item) // Looping through each element in the 'item' array
{
Console.Write(i.ToString() + " "); // Outputting each element followed by a space
}
}
// Method to remove all occurrences of '5' from the array
static int[] test(int[] numbers)
{
int size = numbers.Length; // Storing the size of the input array
int index = 0; // Initializing an index variable to track positions in a new array
int[] arra1 = new int[size]; // Creating a new array to store the modified elements
for (int i = 0; i < size; i++) // Looping through the input array
{
if (numbers[i] != 5) // Checking if the current element is not equal to '5'
{
arra1[index++] = numbers[i]; // Storing non-'5' elements in the new array
}
}
return arra1; // Returning the modified array
}
}
}
Sample Output:
New array: 1 2 3 7 9 11 0 0 0
Flowchart:
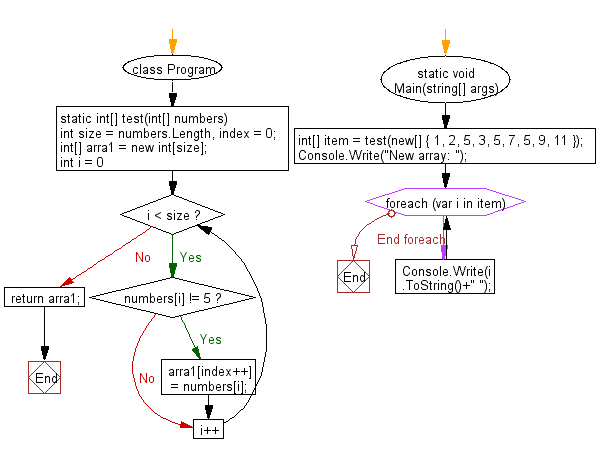
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array from a given array of integers and shifting all zeros to left direction.
Next: Write a C# Sharp program to create new array from a given array of integers shifting all even numbers before all odd numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.