C#: Create new array from a given array of integers shifting all even numbers before all odd numbers
Shift Even Numbers Before Odd Numbers
Write a C# Sharp program to create an array from a given array of integers. Shift all even numbers before all odd numbers.
Visual Presentation:
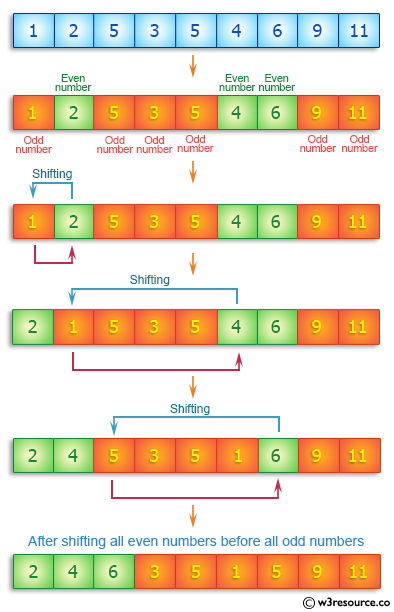
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with a given integer array
// and storing the modified array in 'item'
int[] item = test(new[] { 1, 2, 5, 3, 5, 4, 6, 9, 11 });
// Outputting the elements of the modified array
Console.Write("New array: ");
foreach (var i in item) // Looping through each element in the 'item' array
{
Console.Write(i.ToString()+" "); // Outputting each element followed by a space
}
}
// Method to rearrange the array such that even elements come before odd elements
static int[] test(int[] numbers)
{
int index = 0; // Initializing an index variable to track even numbers' positions
for (int i = 0; i < numbers.Length; i++) // Looping through the input array
{
if (numbers[i] % 2 == 0) // Checking if the current element is even
{
int temp = numbers[index]; // Swapping even and odd elements using a temp variable
numbers[index] = numbers[i];
numbers[i] = temp;
index++; // Incrementing the index to track the next even number position
}
}
return numbers; // Returning the modified array
}
}
}
Sample Output:
New array: 2 4 6 3 5 1 5 9 11
Flowchart:
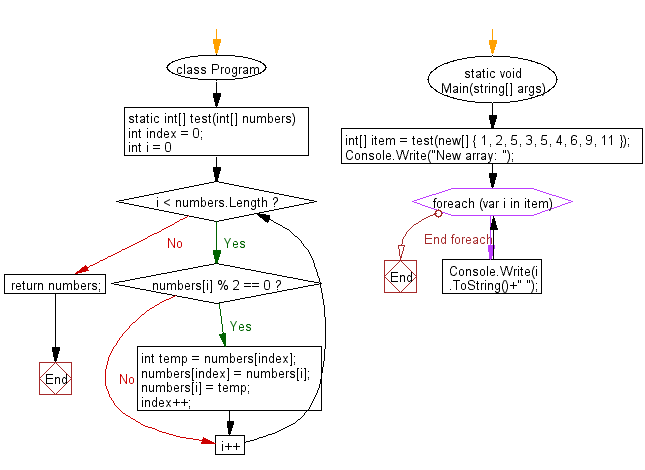
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array after replacing all the values 5 with 0 shifting all zeros to right direction.
Next: Write a C# Sharp program to check if the value of each element is equal or greater than the value of previous element of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.