C#: Check whether the value of each element is equal or greater than the value of previous element of a given array of integers
Check If Each Element ≥ Previous
Write a C# Sharp program to check if the value of each element is equal or greater than the value of the previous element in a given array of integers.
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Outputting the result of the 'test' method with various integer arrays
Console.WriteLine(test(new[] { 5, 5, 1, 5, 5 }));
Console.WriteLine(test(new[] { 1, 2, 3, 4 }));
Console.WriteLine(test(new[] { 3, 3, 5, 5, 5, 5 }));
Console.WriteLine(test(new[] { 1, 5, 5, 7, 8, 10 }));
}
// Method to check if an array is sorted in non-decreasing order
static bool test(int[] numbers)
{
for (int i = 0; i < numbers.Length - 1; i++) // Loop through the array
{
if (numbers[i + 1] < numbers[i]) // Checking if the next element is less than the current element
{
return false; // If so, the array is not sorted, return false
}
}
return true; // If the loop completes without finding elements out of order, return true
}
}
}
Sample Output:
False True True True
Flowchart:
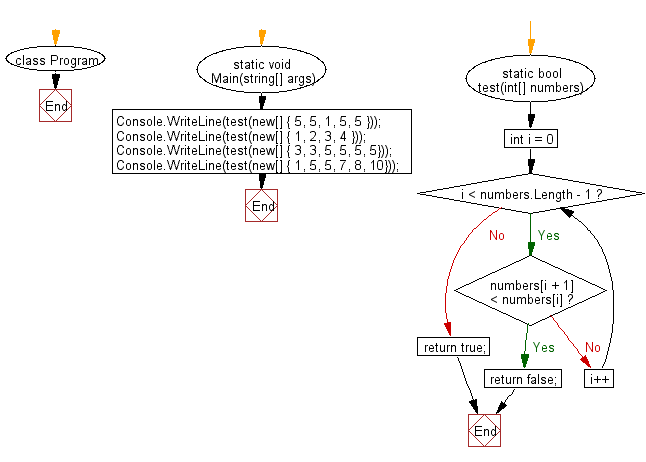
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create new array from a given array of integers shifting all even numbers before all odd numbers.
Next: Write a C# Sharp program to check a given array (length will be atleast 2) of integers and return true if there are two values 15, 15 next to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.