C#: Create a new array using the first n strings from a given array of strings
C# Sharp Basic Algorithm: Exercise-137 with Solution
First n Strings from String Array
Write a C# Sharp program to create an array using the first n strings from a given array of strings. (n>=1 and <=length of the array)
Visual Presentation:
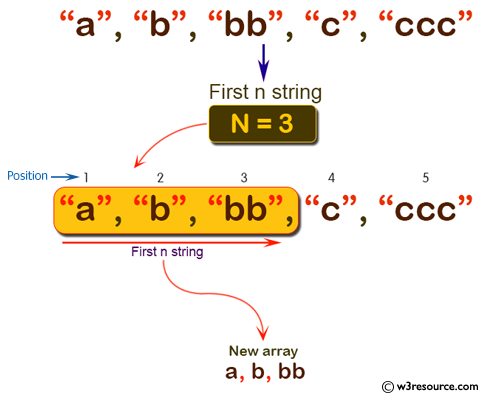
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections; // Importing collections for non-generic interfaces
using System.Collections.Generic; // Importing collections for generic interfaces
using System.Linq; // Importing LINQ for querying collections
using System.Text; // Importing classes for handling strings
using System.Threading.Tasks; // Importing classes for handling asynchronous tasks
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Declaring and initializing a string array 'item' using the 'test' method
string[] item = test(new[] {"a", "b", "bb", "c", "ccc" }, 3);
// Displaying a message to indicate the new array elements
Console.Write("New array: ");
// Iterating through each element in the 'item' array and displaying it
foreach(var i in item)
{
Console.Write(i.ToString() + " ");
}
}
// Method to create a new array with the first 'n' elements from the input array
static string[] test(string[] arr_str, int n)
{
string[] new_array = new string[n]; // Initializing a new string array of size 'n'
// Copying the first 'n' elements from the input array to the new array
for (int i = 0; i < n; i++)
{
new_array[i] = arr_str[i];
}
return new_array; // Returning the new array containing the first 'n' elements
}
}
}
Sample Output:
New array: a b bb
Flowchart:
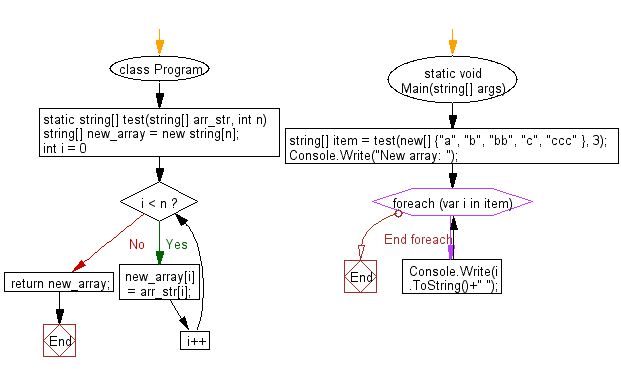
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to count the number of strings with given length in given array of strings.
Next: Write a C# Sharp program to create a new array from a given array of strings using all the strings whose length are matched with given string length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-137.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics