C#: Create a new array from a given array of strings using all the strings whose length are matched with given string length
Strings Matching Given Length
Write a C# Sharp program to create an array from a given array of strings. This is done using all the strings whose lengths matches given string length.
Visual Presentation:
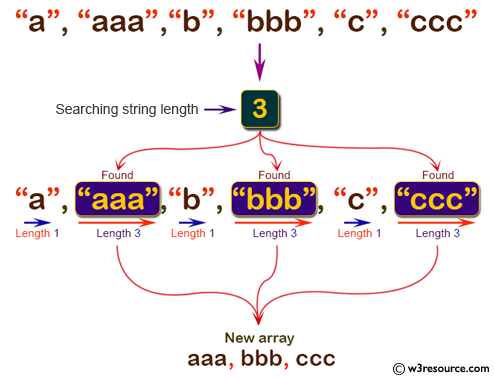
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections; // Importing collections for non-generic interfaces
using System.Collections.Generic; // Importing collections for generic interfaces
using System.Linq; // Importing LINQ for querying collections
using System.Text; // Importing classes for handling strings
using System.Threading.Tasks; // Importing classes for handling asynchronous tasks
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Declaring and initializing an ArrayList 'item' using the 'test' method
ArrayList item = test(new[] {"a", "aaa", "b", "bbb", "c", "ccc" }, 3);
// Displaying a message to indicate the new array elements
Console.Write("New array: ");
// Iterating through each element in the 'item' ArrayList and displaying it
foreach(var i in item)
{
Console.Write(i.ToString() + " ");
}
}
// Method to filter strings of length 'n' from the input array and store them in an ArrayList
static ArrayList test(string[] arr_str, int n)
{
ArrayList result_arra = new ArrayList(); // Initializing a new ArrayList
// Looping through the input string array and adding strings of length 'n' to the ArrayList
for (int i = 0; i < arr_str.Length; i++)
{
if (arr_str[i].Length == n) // Checking if the length of the string matches 'n'
{
result_arra.Add(arr_str[i]); // Adding the string to the ArrayList
}
}
return result_arra; // Returning the ArrayList containing strings of length 'n'
}
}
}
Sample Output:
New array: aaa bbb ccc
Flowchart:
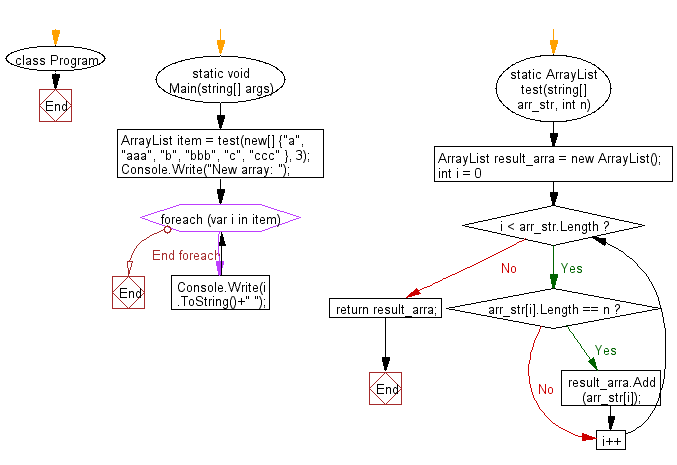
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array using the first n strings from a given array of strings. (n>=1 and <=length of the array)
Next: Write a C# Sharp program to check a positive integer and return true if it contains a number 2.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.