C#: Check a positive integer and return true if it contains a number 2
C# Sharp Basic Algorithm: Exercise-139 with Solution
Check If Number Contains 2
Write a C# Sharp program to check a positive integer and return true if it contains a number 2.
Visual Presentation:
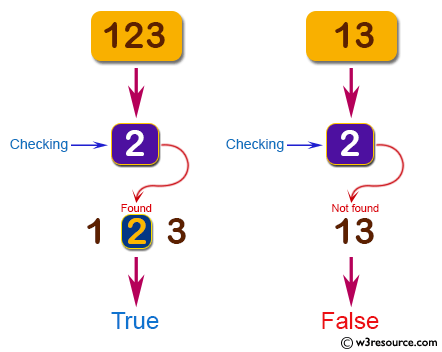
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
using System.Collections; // Importing collections for non-generic interfaces
using System.Collections.Generic; // Importing collections for generic interfaces
using System.Linq; // Importing LINQ for querying collections
using System.Text; // Importing classes for handling strings
using System.Threading.Tasks; // Importing classes for handling asynchronous tasks
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Output the result of the 'test' method with different integer values
Console.WriteLine(test(123));
Console.WriteLine(test(13));
Console.WriteLine(test(222));
}
// Method to check if an integer contains the digit '2'
public static bool test(int n)
{
while (n > 0) // Loop through each digit of the input integer 'n'
{
if (n % 10 == 2) return true; // Check if the last digit is equal to '2'
n /= 10; // Remove the last digit by dividing 'n' by 10
}
return false; // Return false if '2' is not found in 'n'
}
}
}
Sample Output:
True False True
Flowchart:
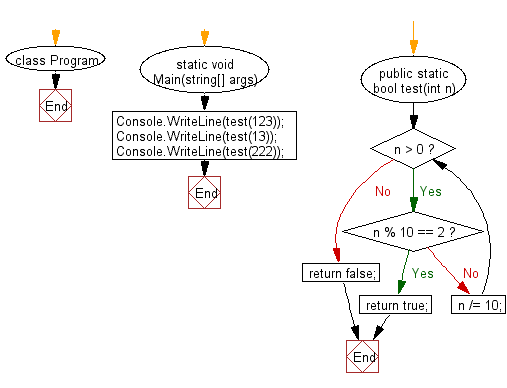
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to create a new array from a given array of strings using all the strings whose length are matched with given string length.
Next: Write a C# Sharp program to create a new array of given length using the odd numbers from a given array of positive integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/csharp-exercises/basic-algo/csharp-basic-algorithm-exercises-139.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics