C#: Create a new array of given length using the odd numbers from a given array of positive integers
Array of Odd Numbers from Given Length
Write a C# Sharp program to create an array of given length using odd numbers from a given array of positive integers.
Visual Presentation:
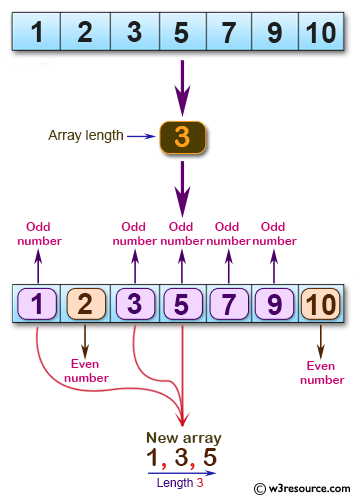
Sample Solution:
C# Sharp Code:
using System; // Importing the System namespace for basic functionality
namespace exercises // Defining a namespace called 'exercises'
{
class Program // Defining a class named 'Program'
{
static void Main(string[] args) // The entry point of the program
{
// Calling the 'test' method with an array and a count value
int[] item = test(new[] { 1, 2, 3, 5, 7, 9, 10 }, 3);
// Displaying the new array elements in the console
Console.Write("New array: ");
foreach (var i in item)
{
Console.Write(i.ToString() + " "); // Output each element followed by a space
}
}
// Method that returns an array of specified count of odd numbers from the input array
public static int[] test(int[] nums, int count)
{
int[] odds = new int[count]; // Initializing a new array to store odd numbers
int j = 0; // Initializing a counter for the 'odds' array
// Loop through the 'nums' array to find and store 'count' number of odd numbers
for (int i = 0; j < count; i++)
{
// Check if the number at 'i' index in 'nums' array is odd
if (nums[i] % 2 != 0)
{
odds[j] = nums[i]; // Store the odd number in the 'odds' array
j++; // Increment the counter to track the number of odd elements found
}
}
return odds; // Return the array containing 'count' number of odd numbers
}
}
}
Sample Output:
New array: 1 3 5
Flowchart:
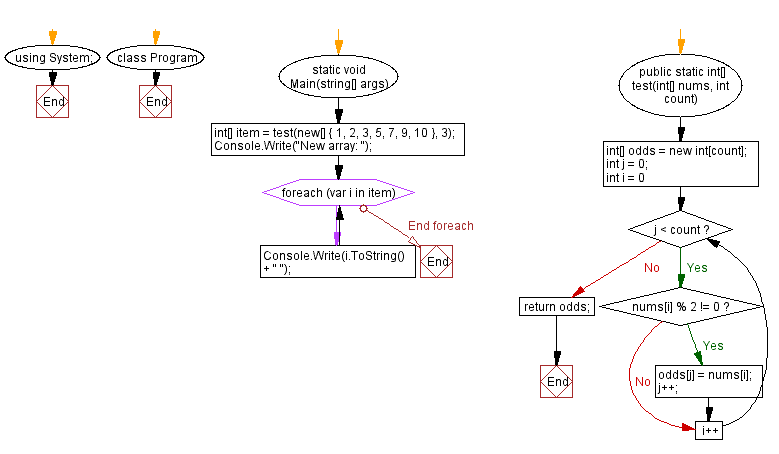
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to check a positive integer and return true if it contains a number 2.
Next: Write a C# Sharp program to create a new list from a given list of integers where each element is multiplied by 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.